インテルにより適用された OpenVINO と複数インテルのハードウェアを使用した Stable Diffusion v2.1¶
この Jupyter ノートブックは、ローカルへのインストール後にのみ起動できます。
このノートブックは、さまざまなハードウェアのさまざまな精度モデルでパフォーマンスを確認する方法を示します。このノートブックは、Optimum-Intel-OpenVINO の使用例を説明するために作成されたものであり、複数回実行するようには最適化されていません。
目次¶
Optimum Intel は、インテル® アーキテクチャー上のエンドツーエンドのパイプラインを高速化するため、Transformers および Diffuser ライブラリーと、インテルが提供するさまざまなツールとライブラリー間のインターフェイスです。詳細については、このリポジトリーをご覧ください。
Note: We suggest you to create a different environment and run the following installation command there.
%pip install -q "optimum-intel[openvino,diffusers]@git+https://github.com/huggingface/optimum-intel.git" "ipywidgets" "transformers>=4.33.0" --extra-index-url https://download.pytorch.org/whl/cpu
import warnings
warnings.filterwarnings('ignore')
利用可能なデバイスの情報を表示¶
available_devices
プロパティーには、システム内で使用可能なデバイスが表示されます。ie.get_property()
の “FULL_DEVICE_NAME” オプションはデバイスの名前を表示します。個別 GPU の ID 名を確認します。統合 GPU (iGPU) と専用 GPU (dGPU) がある場合、iGPU の場合は device_name="GPU.0"
、dGPU の場合は device_name="GPU.1"
と表示されます。"GPU"
に割り当てられる iGPU または dGPU のいずれかのみがある場合。
注: OpenVINO を使用した GPU の詳細については、このリンクを参照してください。Ubuntu* 20.04 または Windows* 11 で問題が発生した場合は、このブログをご覧ください。
from openvino.runtime import Core
ie = Core()
devices = ie.available_devices
for device in devices:
device_name = ie.get_property(device, "FULL_DEVICE_NAME")
print(f"{device}: {device_name}")
CPU: Intel(R) Xeon(R) Gold 6348 CPU @ 2.60GHz
GPU: Intel(R) Data Center GPU Flex 170 (dGPU)
StableDiffusionPipeline
を使用した CPU で完全精度モデルを使用¶
from diffusers import StableDiffusionPipeline
import gc
model_id = "stabilityai/stable-diffusion-2-1-base"
pipe = StableDiffusionPipeline.from_pretrained(model_id)
pipe.save_pretrained("./stabilityai_cpu")
prompt = "red car in snowy forest"
output_cpu = pipe(prompt, num_inference_steps=17).images[0]
output_cpu.save("image_cpu.png")
output_cpu
Fetching 13 files: 0%| | 0/13 [00:00<?, ?it/s]
Downloading model.safetensors: 0%| | 0.00/1.36G [00:00<?, ?B/s]
Downloading (…)ch_model.safetensors: 0%| | 0.00/335M [00:00<?, ?B/s]
Downloading (…)ch_model.safetensors: 0%| | 0.00/3.46G [00:00<?, ?B/s]
0%| | 0/17 [00:00<?, ?it/s]
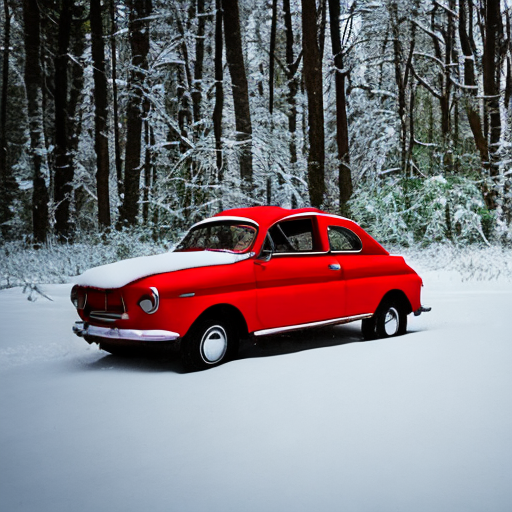
del pipe
gc.collect()
OVStableDiffusionPipeline
を使用した CPU で完全精度モデルを使用¶
from optimum.intel.openvino import OVStableDiffusionPipeline
model_id = "stabilityai/stable-diffusion-2-1-base"
ov_pipe = OVStableDiffusionPipeline.from_pretrained(model_id, export=True, compile=False)
ov_pipe.reshape(batch_size=1, height=512, width=512, num_images_per_prompt=1)
ov_pipe.save_pretrained("./openvino_ir")
ov_pipe.compile()
Framework not specified. Using pt to export to ONNX.
Keyword arguments {'subfolder': '', 'config': {'_class_name': 'StableDiffusionPipeline', '_diffusers_version': '0.10.0.dev0', 'feature_extractor': ['transformers', 'CLIPImageProcessor'], 'requires_safety_checker': False, 'safety_checker': [None, None], 'scheduler': ['diffusers', 'PNDMScheduler'], 'text_encoder': ['transformers', 'CLIPTextModel'], 'tokenizer': ['transformers', 'CLIPTokenizer'], 'unet': ['diffusers', 'UNet2DConditionModel'], 'vae': ['diffusers', 'AutoencoderKL']}} are not expected by StableDiffusionPipeline and will be ignored.
Using framework PyTorch: 2.0.1+cu117
============= Diagnostic Run torch.onnx.export version 2.0.1+cu117 =============
verbose: False, log level: Level.ERROR
======================= 0 NONE 0 NOTE 0 WARNING 0 ERROR ========================
Using framework PyTorch: 2.0.1+cu117
Saving external data to one file...
============= Diagnostic Run torch.onnx.export version 2.0.1+cu117 =============
verbose: False, log level: Level.ERROR
======================= 0 NONE 0 NOTE 0 WARNING 0 ERROR ========================
Using framework PyTorch: 2.0.1+cu117
Using framework PyTorch: 2.0.1+cu117
============= Diagnostic Run torch.onnx.export version 2.0.1+cu117 =============
verbose: False, log level: Level.ERROR
======================= 0 NONE 0 NOTE 0 WARNING 0 ERROR ========================
============= Diagnostic Run torch.onnx.export version 2.0.1+cu117 =============
verbose: False, log level: Level.ERROR
======================= 0 NONE 0 NOTE 0 WARNING 0 ERROR ========================
Compiling the text_encoder...
Compiling the vae_decoder...
Compiling the unet...
prompt = "red car in snowy forest"
output_cpu_ov = ov_pipe(prompt, num_inference_steps=17).images[0]
output_cpu_ov.save("image_ov_cpu.png")
output_cpu_ov
0%| | 0/18 [00:00<?, ?it/s]
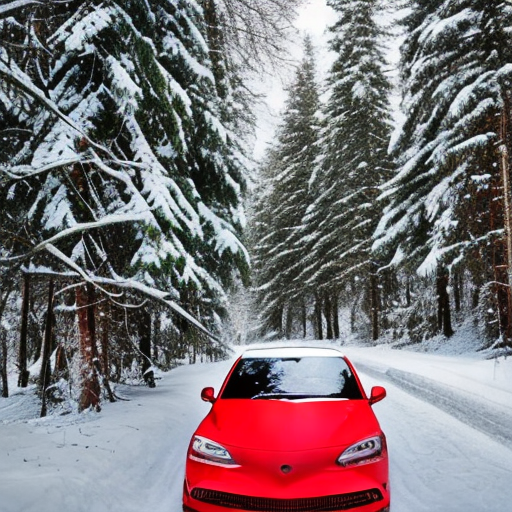
OVStableDiffusionPipeline
を使用した dCPU で完全精度モデルを使用¶
このノートブックのモデルは FP32 精度です。また、OpenVINO 2023.0 の新機能により、GPU で推論を実行するのにモデルを FP16 に変換する必要はありません。
ov_pipe.to("GPU")
ov_pipe.compile()
Compiling the text_encoder...
Compiling the vae_decoder...
Compiling the unet...
prompt = "red car in snowy forest"
output_gpu_ov = ov_pipe(prompt, num_inference_steps=17).images[0]
output_gpu_ov.save("image_ov_gpu.png")
output_gpu_ov
del ov_pipe
gc.collect()