TensorFlow フレームワークを使用した NNCF による量子化対応トレーニング#
この Jupyter ノートブックはオンラインで起動でき、ブラウザーのウィンドウで対話型環境を開きます。ローカルにインストールすることもできます。次のオプションのいずれかを選択します:
このノートブックの目的は、ニューラル・ネットワーク圧縮フレームワーク NNCF 8 ビット量子化を使用して、OpenVINO™ ツールキットによる推論用に TensorFlow モデルを最適化する方法を示すことです。最適化プロセスには次の手順が含まれます:
オリジナルの
FP32
モデルをINT8
に変換します。微調整を使用して精度を復元します。
最適化されたオリジナルのモデルを Frozen Graph にエクスポートし、次に OpenVINO にエクスポートします。
モデルのパフォーマンスを測定および比較します。
さらに高度な使用法については、これらの例を参照してください。
このチュートリアルでは、Imagenette データセットを持つ ResNet-18 モデルを使用します。Imagenette は、ImageNet データセットから簡単に分類された 10 個のクラスのサブセットです。より小さいモデルとデータセットを使用すると、トレーニングとダウンロード時間が短縮されます。
目次:
インポートと設定#
NNCF とすべての補助パッケージを Python コードからインポートします。モデルの名前、入力画像サイズ、使用するバッチサイズ、学習率を設定します。また、モデルの Frozen Graph および OpenVINO IR バージョンが保存されるパスを定義します。
注: チュートリアルを簡略化するために、エラーレベル未満のすべての NNCF ログメッセージ (情報および警告) が無効になっています。運用環境で使用する場合は、
set_log_level(logging.ERROR)
を削除してログを有効にすることを推奨します。
%pip install -q "openvino>=2024.0.0" "nncf>=2.9.0"
%pip install -q "tensorflow-macos>=2.5,<=2.12.0; sys_platform == 'darwin' and platform_machine == 'arm64'"
%pip install -q "tensorflow>=2.5,<=2.12.0; sys_platform == 'darwin' and platform_machine != 'arm64'" # macOS x86
%pip install -q "tensorflow>=2.5,<=2.12.0; sys_platform != 'darwin'"
%pip install -q "tensorflow-datasets>=4.9.0,<4.9.3; platform_system=='Windows'"
%pip install -q "tensorflow-datasets>=4.9.0"
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
from pathlib import Path
import logging
import tensorflow as tf
import tensorflow_datasets as tfds
from nncf import NNCFConfig
from nncf.tensorflow.helpers.model_creation import create_compressed_model
from nncf.tensorflow.initialization import register_default_init_args
from nncf.common.logging.logger import set_log_level
import openvino as ov
set_log_level(logging.ERROR)
MODEL_DIR = Path("model")
OUTPUT_DIR = Path("output")
MODEL_DIR.mkdir(exist_ok=True)
OUTPUT_DIR.mkdir(exist_ok=True)
BASE_MODEL_NAME = "ResNet-18"
fp32_h5_path = Path(MODEL_DIR / (BASE_MODEL_NAME + "_fp32")).with_suffix(".h5")
fp32_ir_path = Path(OUTPUT_DIR / "saved_model").with_suffix(".xml")
int8_pb_path = Path(OUTPUT_DIR / (BASE_MODEL_NAME + "_int8")).with_suffix(".pb")
int8_ir_path = int8_pb_path.with_suffix(".xml")
BATCH_SIZE = 128
IMG_SIZE = (64, 64) # デフォルトの Imagenet 画像サイズ
NUM_CLASSES = 10 # Imagenette データセットの場合
LR = 1e-5
MEAN_RGB = (0.485 * 255, 0.456 * 255, 0.406 * 255) # Imagenet データセットから
STDDEV_RGB = (0.229 * 255, 0.224 * 255, 0.225 * 255) # Imagenet データセットから
fp32_pth_url =
"https://storage.openvinotoolkit.org/repositories/nncf/openvino_notebook_ckpts/305_resnet18_imagenette_fp32_v1.h5"
_ = tf.keras.utils.get_file(fp32_h5_path.resolve(), fp32_pth_url)
print(f"Absolute path where the model weights are saved:\n {fp32_h5_path.resolve()}")
2024-07-13 04:06:54.539917: I tensorflow/core/util/port.cc:110] oneDNN custom operations are on.You may see slightly different numerical results due to floating-point round-off errors from different computation orders.To turn them off, set the environment variable TF_ENABLE_ONEDNN_OPTS=0. 2024-07-13 04:06:54.575556: I tensorflow/core/platform/cpu_feature_guard.cc:182] This TensorFlow binary is optimized to use available CPU instructions in performance-critical operations.To enable the following instructions: AVX2 AVX512F AVX512_VNNI FMA, in other operations, rebuild TensorFlow with the appropriate compiler flags. 2024-07-13 04:06:55.171044: W tensorflow/compiler/tf2tensorrt/utils/py_utils.cc:38] TF-TRT Warning: Could not find TensorRT
INFO:nncf:NNCF initialized successfully.Supported frameworks detected: torch, tensorflow, onnx, openvino
WARNING:nncf:NNCF provides best results with torch==2.15.*, while current torch version is 2.12.0. If you encounter issues, consider switching to torch==2.15.*
Downloading data from
https://storage.openvinotoolkit.org/repositories/nncf/openvino_notebook_ckpts/305_resnet18_imagenette_fp32_v1.h5
134604992/134604992 [==============================] - 1s 0us/step
Absolute path where the model weights are saved: /opt/home/k8sworker/ci-ai/cibuilds/ov-notebook/OVNotebookOps-727/.workspace/scm/ov-notebook/notebooks/tensorflow-quantization-aware-training/model/ResNet-18_fp32.h5
データセットの前処理#
Imagenette 160px データセットをダウンロードして準備します。
クラス数: 10
ダウンロード・サイズ: 94.18 MiB
| Split | Examples |
|--------------|----------|
| 'train' | 12,894 |
| 'validation' | 500 |
datasets, datasets_info = tfds.load(
"imagenette/160px",
shuffle_files=True,
as_supervised=True,
with_info=True,
read_config=tfds.ReadConfig(shuffle_seed=0),
)
train_dataset, validation_dataset = datasets["train"], datasets["validation"]
fig = tfds.show_examples(train_dataset, datasets_info)
2024-07-13 04:07:01.446767: E tensorflow/compiler/xla/stream_executor/cuda/cuda_driver.cc:266] failed call to cuInit:CUDA_ERROR_COMPAT_NOT_SUPPORTED_ON_DEVICE: forward compatibility was attempted on non supported HW 2024-07-13 04:05:446799:I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:168] retrieving CUDA diagnostic information for host: iotg-dev-workstation-07 2024-07-13 04:07:01.446804: I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:175] hostname: iotg-dev-workstation-07 2024-07-13 04:07:01.446954: I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:199] libcuda reported version is: 470.223.2 2024-07-13 04:07:01.446971: I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:203] kernel reported version is: 470.182.3 2024-07-13 04:07:01.446974: E tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:312] kernel version 470.182.3 does not match DSO version 470.223.2 -- cannot find working devices in this configuration 2024-07-13 04:07:01.553632: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1] [[{{node Placeholder/_4}}]] 2024-07-13 04:07:01.553982: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1] [[{{node Placeholder/_4}}]] 2024-07-13 04:07:01.624806: W tensorflow/core/kernels/data/cache_dataset_ops.cc:856] The calling iterator did not fully read the dataset being cached. In order to avoid unexpected truncation of the dataset, the partially cached contents of the dataset will be discarded. This can happen if you have an input pipeline similar to dataset.cache().take(k).repeat(). You should use dataset.take(k).cache().repeat() instead.
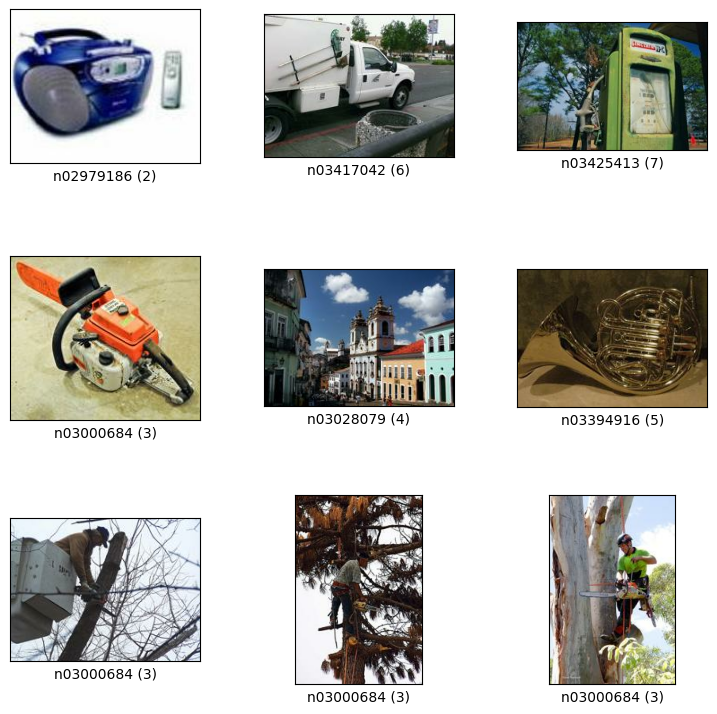
def preprocessing(image, label):
image = tf.image.resize(image, IMG_SIZE)
image = image - MEAN_RGB
image = image / STDDEV_RGB
label = tf.one_hot(label, NUM_CLASSES)
return image, label
train_dataset = train_dataset.map(preprocessing,
num_parallel_calls=tf.data.experimental.AUTOTUNE).batch(BATCH_SIZE).prefetch(tf.data.experimental.AUTOTUNE)
validation_dataset = (
validation_dataset.map(preprocessing,
num_parallel_calls=tf.data.experimental.AUTOTUNE).batch(BATCH_SIZE).prefetch(tf.data.experimental.AUTOTUNE)
)
浮動小数点モデルを定義#
def residual_conv_block(filters, stage, block, strides=(1, 1), cut="pre"):
def layer(input_tensor):
x = tf.keras.layers.BatchNormalization(epsilon=2e-5)(input_tensor)
x = tf.keras.layers.Activation("relu")(x)
# ショートカット接続を定義
if cut == "pre":
shortcut = input_tensor
elif cut == "post":
shortcut = tf.keras.layers.Conv2D(
filters,
(1, 1),
strides=strides,
kernel_initializer="he_uniform",
use_bias=False,
)(x)
# 畳み込みレイヤーを続行
x = tf.keras.layers.ZeroPadding2D(padding=(1, 1))(x)
x = tf.keras.layers.Conv2D(
filters,
(3, 3),
strides=strides,
kernel_initializer="he_uniform",
use_bias=False,
)(x)
x = tf.keras.layers.BatchNormalization(epsilon=2e-5)(x)
x = tf.keras.layers.Activation("relu")(x)
x = tf.keras.layers.ZeroPadding2D(padding=(1, 1))(x)
x = tf.keras.layers.Conv2D(filters, (3, 3), kernel_initializer="he_uniform", use_bias=False)(x)
# 残余接続を追加
x = tf.keras.layers.Add()([x, shortcut])
return x
return layer
def ResNet18(input_shape=None):
"""Instantiates the ResNet18 architecture."""
img_input = tf.keras.layers.Input(shape=input_shape, name="data")
# ResNet18 ボトム
x = tf.keras.layers.BatchNormalization(epsilon=2e-5, scale=False)(img_input)
x = tf.keras.layers.ZeroPadding2D(padding=(3, 3))(x)
x = tf.keras.layers.Conv2D(64, (7, 7), strides=(2, 2), kernel_initializer="he_uniform", use_bias=False)(x)
x = tf.keras.layers.BatchNormalization(epsilon=2e-5)(x)
x = tf.keras.layers.Activation("relu")(x)
x = tf.keras.layers.ZeroPadding2D(padding=(1, 1))(x)
x = tf.keras.layers.MaxPooling2D((3, 3), strides=(2, 2), padding="valid")(x)
# ResNet18 ボディー
repetitions = (2, 2, 2, 2)
for stage, rep in enumerate(repetitions):
for block in range(rep):
filters = 64 * (2**stage)
if block == 0 and stage == 0:
x = residual_conv_block(filters, stage, block, strides=(1, 1), cut="post")(x)
elif block == 0:
x = residual_conv_block(filters, stage, block, strides=(2, 2), cut="post")(x)
else:
x = residual_conv_block(filters, stage, block, strides=(1, 1), cut="pre")(x)
x = tf.keras.layers.BatchNormalization(epsilon=2e-5)(x)
x = tf.keras.layers.Activation("relu")(x)
# ResNet18 トップ
x = tf.keras.layers.GlobalAveragePooling2D()(x)
x = tf.keras.layers.Dense(NUM_CLASSES)(x)
x = tf.keras.layers.Activation("softmax")(x)
# モデルを作成
model = tf.keras.models.Model(img_input, x)
return model
IMG_SHAPE = IMG_SIZE + (3,)
fp32_model = ResNet18(input_shape=IMG_SHAPE)
浮動小数点モデルの事前トレーニング#
モデル圧縮に NNCF を使用する場合、ユーザーが事前トレーニングされたモデルとトレーニング・パイプラインを持っていることを前提としています。
注 チュートリアルを簡単にするため、
FP32
モデルのトレーニングをスキップして、提供されている重みをロードすることを推奨します。
# 浮動小数点の重みをロード
fp32_model.load_weights(fp32_h5_path)
# 浮動小数点モデルをコンパイル
fp32_model.compile(.compile(
loss=tf.keras.losses.CategoricalCrossentropy(label_smoothing=0.1),
metrics=[tf.keras.metrics.CategoricalAccuracy(name="acc@1")],
)
# 浮動小数点モデルを検証
test_loss, acc_fp32 = fp32_model.evaluate(
validation_dataset,
callbacks=tf.keras.callbacks.ProgbarLogger(stateful_metrics=["acc@1"]),
)
print(f"\nAccuracy of FP32 model: {acc_fp32:.3f}")
2024-07-13 04:07:02.579874: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_0' with dtype string and shape [1]
[[{{node Placeholder/_0}}]]
2024-07-13 04:07:02.580249: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_2' with dtype string and shape [1]
[[{{node Placeholder/_2}}]]
4/4 [==============================] - 1s 288ms/sample - loss: 0.9807 - acc@1: 0.8220
Accuracy of FP32 model: 0.822
量子化の作成と初期#
NNCF は、通常のトレーニング・パイプラインに統合することで、圧縮を意識したトレーニングを可能にします。このフレームワークは、元のトレーニング・コードへの変更が最小限になるように設計されています。量子化は最も単純なシナリオであり、わずか 3 つの変更で済みます。
NNCF パラメーターを設定して圧縮を指定します
nncf_config_dict = {
"input_info": {"sample_size": [1, 3] + list(IMG_SIZE)},
"log_dir": str(OUTPUT_DIR), # NNCF 固有のログ出力のログ・ディレクトリー
"compression": {
"algorithm": "quantization", # ここでアルゴリズムを指定 },
}
nncf_config = NNCFConfig.from_dict(nncf_config_dict)
量子化範囲の値を初期化し、指定された数のサンプルを使用して、収集された統計からどの活性化を署名するか署名しないかを決定するデータローダーを提供します。
nncf_config = register_default_init_args(nncf_config=nncf_config,
data_loader=train_dataset, batch_size=BATCH_SIZE)
事前トレーニングされた
FP32
モデルと構成オブジェクトから、圧縮の微調整が可能なラップされたモデルを作成します。
compression_ctrl, int8_model = create_compressed_model(fp32_model, nncf_config)
2024-07-13 04:07:05.362029: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_1' with dtype string and shape [1] [[{{node Placeholder/_1}}]] 2024-07-13 04:07:05.362414: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_2' with dtype string and shape [1] [[{{node Placeholder/_2}}]] 2024-07-13 04:07:06.318865: W tensorflow/core/kernels/data/cache_dataset_ops.cc:856] The calling iterator did not fully read the dataset being cached. In order to avoid unexpected truncation of the dataset, the partially cached contents of the dataset will be discarded.This can happen if you have an input pipeline similar to dataset.cache().take(k).repeat().You should use dataset.take(k).cache().repeat() instead. 2024-07-13 04:07:07.067794: W tensorflow/core/kernels/data/cache_dataset_ops.cc:856] The calling iterator did not fully read the dataset being cached.In order to avoid unexpected truncation of the dataset, the partially cached contents of the dataset will be discarded.This can happen if you have an input pipeline similar to dataset.cache().take(k).repeat().You should use dataset.take(k).cache().repeat() instead. 2024-07-13 04:07:15.330371: W tensorflow/core/kernels/data/cache_dataset_ops.cc:856] The calling iterator did not fully read the dataset being cached.In order to avoid unexpected truncation of the dataset, the partially cached contents of the dataset will be discarded.This can happen if you have an input pipeline similar to dataset.cache().take(k).repeat().You should use dataset.take(k).cache().repeat() instead.
量子化の初期化後、検証セットで新しいモデルを評価します。ここで実証されているような単純なケースでは、精度は浮動小数点 FP32
モデルの精度とそれほど変わらないはずです。
# INT8 モデルをコンパイル
Int8_model.compile(.compile(
optimizer=tf.keras.optimizers.Adam(learning_rate=LR),
loss=tf.keras.losses.CategoricalCrossentropy(label_smoothing=0.1),
metrics=[tf.keras.metrics.CategoricalAccuracy(name="acc@1")],
)
# INT8 モデルを検証
test_loss, test_acc = int8_model.evaluate(
validation_dataset,
callbacks=tf.keras.callbacks.ProgbarLogger(stateful_metrics=["acc@1"]),
)
4/4 [==============================] - 1s 303ms/sample - loss: 0.9766 - acc@1: 0.8120
圧縮モデルを微調整#
このステップでは、量子化モデルの精度をさらに向上させるため定期的な微調整プロセスが適用されます。通常、元のモデルのトレーニングの最後に使用されるのと同じ学習率を小さくして、数エポックの調整が必要になります。トレーニング・パイプラインにその他の変更は必要ありません。以下に簡単な例を示します。
print(f"\nAccuracy of INT8 model after initialization: {test_acc:.3f}")
# INT8 モデルをトレーニング
int8_model.fit(train_dataset, epochs=2)
# NT8 モデルを検証
test_loss, acc_int8 = int8_model.evaluate(
validation_dataset,
callbacks=tf.keras.callbacks.ProgbarLogger(stateful_metrics=["acc@1"]),
)
print(f"\nAccuracy of INT8 model after fine-tuning: {acc_int8:.3f}")
print(f"\nAccuracy drop of tuned INT8 model over pre-trained FP32 model: {acc_fp32 - acc_int8:.3f}")
Accuracy of INT8 model after initialization: 0.812
Epoch 1/2
101/101 [==============================] - 49s 415ms/step - loss: 0.7134 - acc@1: 0.9299
Epoch 2/2
101/101 [==============================] - 42s 413ms/step - loss: 0.6807 - acc@1: 0.9489
4/4 [==============================] - 1s 139ms/sample - loss: 0.9760 - acc@1: 0.8160
Accuracy of INT8 model after fine-tuning: 0.816
Accuracy drop of tuned INT8 model over pre-trained FP32 model: 0.006
OpenVINO 中間表現 (IR) へのモデルのエクスポート#
モデル変換 Python API を使用して、モデルを OpenVINO IR に変換します。
モデル変換の詳細については、このページを参照してください。
このコマンドの実行には時間がかかる場合があります。
model_ir_fp32 = ov.convert_model(fp32_model)
WARNING:tensorflow:Please fix your imports.Module tensorflow.python.training.tracking.base has been moved to tensorflow.python.trackable.base.The old module will be deleted in version 2.11.
WARNING:tensorflow:Please fix your imports.Module tensorflow.python.training.tracking.base has been moved to tensorflow.python.trackable.base.The old module will be deleted in version 2.11.
model_ir_int8 = ov.convert_model(int8_model)
ov.save_model(model_ir_fp32, fp32_ir_path, compress_to_fp16=False)
ov.save_model(model_ir_int8, int8_ir_path, compress_to_fp16=False)
推論時間の計算によるベンチマーク・モデルのパフォーマンス#
最後に、ベンチマーク・ツールを使用して、FP32
モデルと INT8
モデルの推論パフォーマンスを測定します。
- OpenVINO の推論パフォーマンス測定ツール。デフォルトでは、ベンチマーク・ツールは CPU 上の非同期モードで推論を 60 秒間実行します。推論速度をレイテンシー (画像あたりのミリ秒) およびスループット (1 秒あたりのフレーム数) の値として返します。
注: このノートブックは、
benchmark_app
を 15 秒間実行して、パフォーマンスを簡単に示します。より正確なパフォーマンスを得るには、他のアプリケーションを閉じて、ターミナル/コマンドプロンプトでbenchmark_app
を実行することを推奨します。benchmark_app -m model.xml -d CPU
を実行して、CPU で非同期推論のベンチマークを 1 分間実行します。GPU でベンチマークを行うには、CPU を GPU に変更します。benchmark_app --help
を実行すると、すべてのコマンドライン・オプションの概要が表示されます。
ドロップダウン・リストからベンチマーク・デバイスを選択してください:
import ipywidgets as widgets
# OpenVINO ランタイムを初期化
core = ov.Core()
device = widgets.Dropdown(
options=core.available_devices,
value="CPU",
description="Device:", disabled=False,
)
device
Dropdown(description='Device:', options=('CPU',), value='CPU')
def parse_benchmark_output(benchmark_output):
parsed_output = [line for line in benchmark_output if "FPS" in line]
print(*parsed_output, sep="\n")
print("Benchmark FP32 model (IR)")
benchmark_output = ! benchmark_app -m $fp32_ir_path -d $device.value -api async -t 15 -shape [1,64,64,3]
parse_benchmark_output(benchmark_output)
print("\nBenchmark INT8 model (IR)")
benchmark_output = ! benchmark_app -m $int8_ir_path -d $device.value -api async -t 15 -shape [1,64,64,3]
parse_benchmark_output(benchmark_output)
Benchmark FP32 model (IR)
[ INFO ] Throughput: 2839.00 FPS
Benchmark INT8 model (IR)
[ INFO ] Throughput: 11068.25 FPS
参考としてデバイス情報を表示します。
core = ov.Core()
core.get_property(device.value, "FULL_DEVICE_NAME")
'Intel(R) Core(TM) i9-10920X CPU @ 3.50GHz'