OpenVINO と Qwen-Agent を使用して関数呼び出しエージェントを作成#
この Jupyter ノートブックは、ローカルへのインストール後にのみ起動できます。
LLM は、トレーニングされた知識とコンテキストとして提供される追加の知識に限定されているため、有用な情報に提供された知識が欠けている場合、モデルは “回り道” をしても他のソースでそれを見つけることができません。これが、エージェントの概念を導入する理由です。
エージェントの主な考え方は、言語モデルを使用して、実行する一連のアクションを選択することです。エージェントは、言語モデルが推論エンジンとして使用され、どのアクションをどの順序で実行するか決定します。エージェントは、LLM によって実行され、検索エンジン、データベース、ウェブサイトなど一連のツールと統合されたアプリケーションとして考えることができます。エージェント内では、LLM は、ユーザー入力に基づいて、要求を満たすために必要な一連のアクションを計画および実行できる推論エンジンです。
エージェント#
Qwen-Agent は、Qwen の命令実行、ツールの使用、計画、およびメモリー機能に基づいて LLM アプリケーションを開発するフレームワークです。また、ブラウザー・アシスタント、コード・インタープリター、カスタム・アシスタントなどのサンプル・アプリケーションも付属しています。
このノートブックでは、OpenVINO と Qwen-Agent を使用して関数呼び出しエージェントを段階的に作成する方法を説明します。
目次:
必要条件#
import os
os.environ["GIT_CLONE_PROTECTION_ACTIVE"] = "false"
%pip install -Uq pip
%pip uninstall -q -y optimum optimum-intel
%pip install --pre -Uq openvino openvino-tokenizers[transformers] --extra-index-url https://storage.openvinotoolkit.org/simple/wheels/nightly
%pip install -q --extra-index-url https://download.pytorch.org/whl/cpu\ "git+https://github.com/huggingface/optimum-intel.git"\
"git+https://github.com/openvinotoolkit/nncf.git"\
"torch>=2.1"\
"datasets"\
"accelerate"\
"qwen-agent>=0.0.6" "transformers>=4.38.1" "gradio==4.21.0", "modelscope-studio>=0.4.0" "langchain>=0.2.3" "langchain-community>=0.2.4" "wikipedia"
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
WARNING: typer 0.12.3 does not provide the extra 'all'
Note: you may need to restart the kernel to use updated packages.
import requests
from PIL import Image
openvino_logo = "openvino_log.png"
url = "https://cdn-avatars.huggingface.co/v1/production/uploads/1671615670447-6346651be2dcb5422bcd13dd.png"
image = Image.open(requests.get(url, stream=True).raw)
image.save(openvino_logo)
関数呼び出しエージェントを作成#
関数呼び出しにより、モデルは 1 つ以上のツールを呼び出すタイミングを検出し、それらのツールに渡す入力で応答できます。API 呼び出しでは、ツールを記述し、モデルがこれらのツールを呼び出すための引数を含む JSON などの構造化オブジェクトをインテリジェントに出力するように選択できます。ツール API の目標は、一般的なテキスト補完 API やチャット API を使用する場合よりも有効で有用なツール呼び出しを確実に返すことです。
この構造化された出力と、複数のツールをツール呼び出しチャットモデルにバインドし、モデルがどのツールを呼び出すかを選択できるという事実を組み合わせて、クエリーが解決されるまでツールを繰り返し呼び出して結果を受け取るエージェントを作成できます。
関数を作成#
まず、現在の天気の情報を取得するサンプル関数/ツールを作成する必要があります。
import json
def get_current_weather(location, unit="fahrenheit"):
"""Get the current weather in a given location"""
if "tokyo" in location.lower():
return json.dumps({"location": "Tokyo", "temperature": "10", "unit": "celsius"})
elif "san francisco" in location.lower():
return json.dumps({"location": "San Francisco", "temperature": "72", "unit": "fahrenheit"})
elif "paris" in location.lower():
return json.dumps({"location": "Paris", "temperature": "22", "unit": "celsius"})
else:
return json.dumps({"location": location, "temperature": "unknown"})
関数の名前と説明を JSON リストにラップすると、LLM が現在のタスクに対してどの関数を呼び出すかを判断するのに役立ちます。
functions = [
{
"name": "get_current_weather",
"description": "Get the current weather in a given location",
"parameters": {
"type": "object",
"properties": {
"location": {
"type": "string",
"description": "The city and state, e.g. San Francisco, CA",
},
"unit": {"type": "string", "enum": ["celsius", "fahrenheit"]},
},
"required": ["location"],
},
}
]
モデルのダウンロード#
大規模言語モデル (LLM) は、エージェントのコア・コンポーネントです。この例では、Qwen-Agent フレームワークで OpenVINO LLM モデルを作成する方法を示します。Qwen2 はテキスト生成中の関数呼び出しをサポートできるため、エージェント・パイプラインの LLM として Qwen/Qwen2-7B-Instruct
を選択します。
Qwen/Qwen2-7B-Instruct - Qwen2 は、Qwen 大規模言語モデルの新しいシリーズです。以前リリースされた Qwen1.5 を含む最先端のオープンソース言語モデルと比較すると、Qwen2 は一般的にほとんどのオープンソース・モデルを上回り、言語理解、言語生成、多言語機能、コーディング、数学、推論などモデルカードを対象とするベンチマークで独自のモデルに対して競争力があることが実証されています。
LLM をローカルで実行するには、最初のステップでモデルをダウンロードする必要があります。CLI からモデルを OpenVINO IR 形式にエクスポートし、ローカルフォルダーからモデルを読み込むことができます。
from pathlib import Path
model_id = "Qwen/Qwen2-7B-Instruct"
model_path = "Qwen2-7B-Instruct-ov"
if not Path(model_path).exists():
!optimum-cli export openvino --model {model_id} --task text-generation-with-past --trust-remote-code --weight-format int4 --ratio 0.72 {model_path}
LLM の推論デバイスを選択#
import openvino as ov
import ipywidgets as widgets
core = ov.Core()
support_devices = core.available_devices
if "NPU" in support_devices:
support_devices.remove("NPU")
device = widgets.Dropdown(
options=support_devices + ["AUTO"],
value="CPU",
description="Device:",
disabled=False,
)
device
Dropdown(description='Device:', options=('CPU', 'AUTO'), value='CPU')
Qwen-Agent 用の LLM を作成#
OpenVINO は Qwen-Agent
フレームワークに統合されました。次の方法で、Qwen-Agent
パイプライン用の OpenVINO ベースの LLM を作成できます。
from qwen_agent.llm import get_chat_model
ov_config = {"PERFORMANCE_HINT": "LATENCY", "NUM_STREAMS": "1", "CACHE_DIR": ""}
llm_cfg = {
"ov_model_dir": model_path,
"model_type": "openvino",
"device": device.value,
"ov_config": ov_config,
# (オプション) 生成用の LLM ハイパーパラメーター:
"generate_cfg": {"top_p": 0.8},
}
llm = get_chat_model(llm_cfg)
Compiling the model to CPU ...
Special tokens have been added in the vocabulary, make sure the associated word embeddings are fine-tuned or trained.
活性化の動的量子化と CPU 上の KV キャッシュ量子化により、推論速度がさらに向上します。これらのオプションは、次のように ov_config
で有効にできます:
ov_config = {
"KV_CACHE_PRECISION": "u8",
"DYNAMIC_QUANTIZATION_GROUP_SIZE": "32",
"PERFORMANCE_HINT": "LATENCY",
"NUM_STREAMS": "1",
"CACHE_DIR": "",
}
関数呼び出しパイプラインを作成#
関数と LLM を定義した後、関数呼び出し機能を備えたエージェント・パイプラインを構築できます。
関数呼び出し#
Qwen2 関数呼び出しのワークフローは、いくつかのステップで構成されます:
リクエストを送信するロール
user
。モデルが関数を呼び出すかどうかを確認し、必要であれば関数を呼び出します。
function
の結果から観測値を取得します。観察結果を
assistant
の最終応答に統合します。
典型的なマルチターンのダイアログ構造は次のとおりです:
クエリー:
{'role': 'user', 'content': 'create a picture of cute cat'},
関数呼び出し:
{'role': 'assistant', 'content': '', 'function_call': {'name': 'my_image_gen', 'arguments': '{"prompt": "a cute cat"}'}},
観察:
{'role': 'function', 'content': '{"image_url": "https://image.pollinations.ai/prompt/a%20cute%20cat"}', 'name': 'my_image_gen'}
最終回答:
{'role': 'assistant', 'content': "Here is the image of a cute cat based on your description:\n\n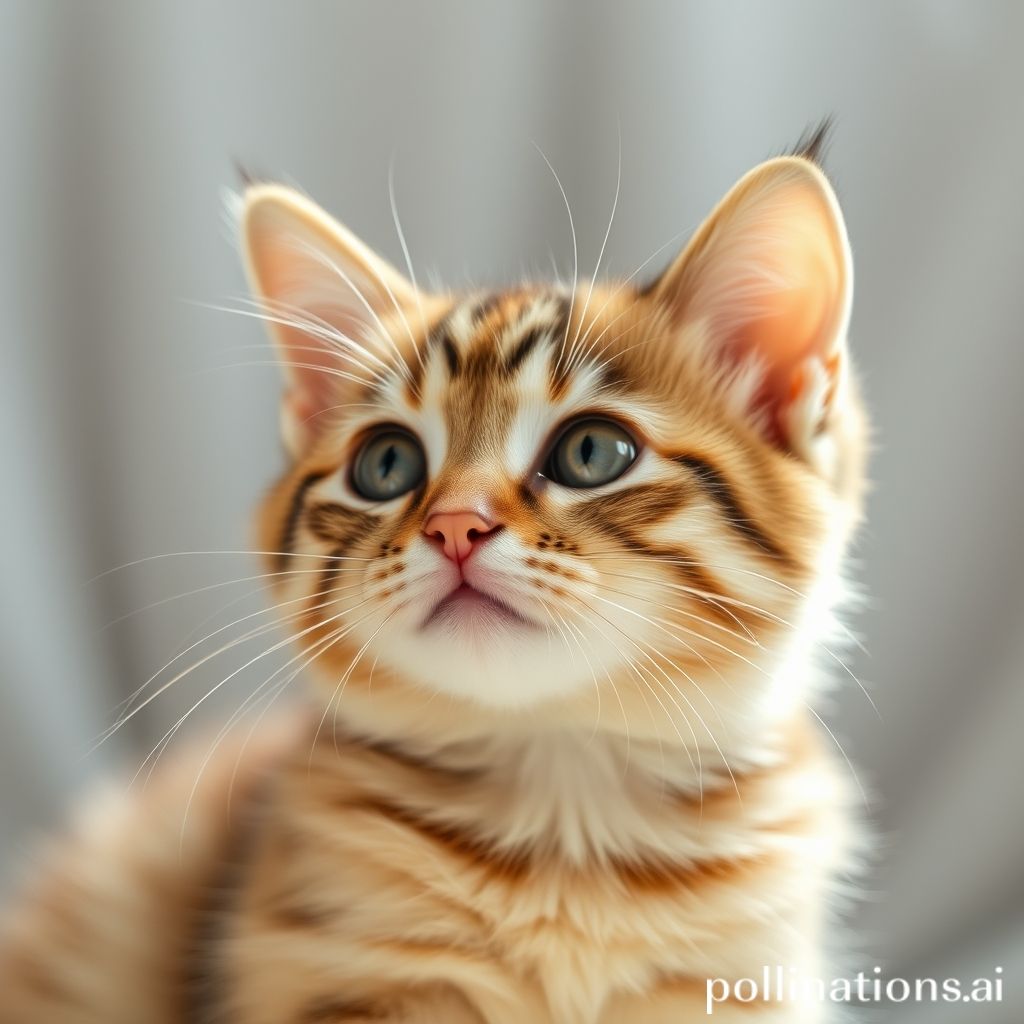."}
print("# User question:")
messages = [{"role": "user", "content": "What's the weather like in San Francisco?"}]
print(messages)
print("# Assistant Response 1:")
responses = []
# ステップ 1: リクエストを送信するロール `user`
responses = llm.chat(
messages=messages,
functions=functions,
stream=False,
)
print(responses)
messages.extend(responses)
# ステップ 2: モデルが関数を呼び出す必要があるかどうかを確認し、必要に応じて関数を呼び出す
last_response = messages[-1]
if last_response.get("function_call", None):
available_functions = {
"get_current_weather": get_current_weather,
} # この例では 1 つの関数のみですが、複数の関数を使用できます
function_name = last_response["function_call"]["name"]
function_to_call = available_functions[function_name]
function_args = json.loads(last_response["function_call"]["arguments"])
function_response = function_to_call(
location=function_args.get("location"),
)
print("# Function Response:")
print(function_response)
# ステップ 3: `function` の結果から観測値を取得
messages.append(
{
"role": "function",
"name": function_name,
"content": function_response,
}
)
print("# Assistant Response 2:")
# ステップ 4: 関数からの観察を最終応答に統合
responses = llm.chat(
messages=messages,
functions=functions,
stream=False,
)
print(responses)
# User question:
[{'role': 'user', 'content': "What's the weather like in San Francisco?"}] # Assistant Response 1: [{'role': 'assistant', 'content': '', 'function_call': {'name': 'get_current_weather', 'arguments': '{"location": "San Francisco, CA"}'}}]
# Function Response:
{"location": "San Francisco", "temperature": "72", "unit": "fahrenheit"}
# Assistant Response 2:
[{'role': 'assistant', 'content': 'The current weather in San Francisco is 72 degrees Fahrenheit.'}]
インタラクティブなデモ#
Gradio を使用してインタラクティブなエージェントを作成してみます。
ツールを作成#
Qwen-Agent はツールを登録するメカニズムを提供します。例えば、独自の画像生成ツールを登録するには、次のようにします:
ツールの名前、説明、パラメーターを指定します。
@register_tool('my_image_gen')
に渡される文字列は、クラスの.name
属性として自動的に追加され、ツールの一意の識別子として機能することに注意してください。call(...)
関数を実装します。
このノートブックでは、例として 3 つのツールを作成します:
image_generation: AI ペイント (画像生成) サービスで、テキストの説明を入力し、テキスト情報に基づいて描画された画像の URL を返します。
get_current_weather: 指定された都市の現在の天気を取得します。
wikipedia: Wikipedia のラッパー。人物、場所、企業、事実、歴史的出来事、その他の主題に関する一般的な質問に答える場合に役立ちます。
import urllib.parse
import json5
import requests
from qwen_agent.tools.base import BaseTool, register_tool
@register_tool("image_generation")
class ImageGeneration(BaseTool):
description = "AI painting (image generation) service, input text description, and return the image URL drawn based on text information."
parameters = [{"name": "prompt", "type": "string", "description": "Detailed description of the desired image content, in English", "required": True}]
def call(self, params: str, **kwargs) -> str:
prompt = json5.loads(params)["prompt"]
prompt = urllib.parse.quote(prompt)
return json5.dumps({"image_url": f"https://image.pollinations.ai/prompt/{prompt}"}, ensure_ascii=False)
@register_tool("get_current_weather")
class GetCurrentWeather(BaseTool):
description = "Get the current weather in a given city name."
parameters = [{"name": "city_name", "type": "string", "description": "The city and state, e.g. San Francisco, CA", "required": True}]
def call(self, params: str, **kwargs) -> str:
# `params` は LLM エージェントによって生成される引数です
city_name = json5.loads(params)["city_name"]
key_selection = {
"current_condition": [
"temp_C",
"FeelsLikeC",
"humidity",
"weatherDesc",
"observation_time",
],
}
resp = requests.get(f"https://wttr.in/{city_name}?format=j1")
resp.raise_for_status()
resp = resp.json()
ret = {k: {_v: resp[k][0][_v] for _v in v} for k, v in key_selection.items()}
return str(ret)
@register_tool("wikipedia")
class Wikipedia(BaseTool):
description = "A wrapper around Wikipedia.Useful for when you need to answer general questions about people, places, companies, facts, historical events, or other subjects."
parameters = [{"name": "query", "type": "string", "description": "Query to look up on wikipedia", "required": True}]
def call(self, params: str, **kwargs) -> str:
# `params` は LLM エージェントによって生成された引数です
from langchain.tools import WikipediaQueryRun
from langchain_community.utilities import WikipediaAPIWrapper
query = json5.loads(params)["query"]
wikipedia = WikipediaQueryRun(api_wrapper=WikipediaAPIWrapper(top_k_results=2, doc_content_chars_max=1000))
resutlt = wikipedia.run(query)
return str(resutlt)
tools = ["image_generation", "get_current_weather", "wikipedia"]
Qwen-Agent と Gradio UI を使用して AI エージェントのデモを作成#
Agent クラスは Qwen-Agent の高レベル・インターフェイスとして機能し、Agent オブジェクトはツール呼び出しと LLM (大規模言語モデル) のインターフェイスを統合します。エージェントは入力としてメッセージのリストを受け取り、メッセージのリストを生成するジェネレーターを生成して、出力メッセージのストリームを効果的に提供します。
Qwen-Agent は汎用エージェント・クラスである Assistant
クラスを提供します。このクラスを直接インスタンス化することで、ほとんどのシングル・エージェント・タスクを処理できます。機能:
ロールプレイングをサポートします。
自動計画およびツール呼び出し機能を提供します。
RAG (検索拡張生成): ドキュメント入力を受け入れ、統合された RAG を使用してドキュメントを解析できます。
from qwen_agent.agents import Assistant
from qwen_agent.gui import WebUI
bot = Assistant(llm=llm_cfg, function_list=tools, name="OpenVINO Agent")
Compiling the model to CPU ...
Special tokens have been added in the vocabulary, make sure the associated word embeddings are fine-tuned or trained.
from typing import List
from qwen_agent.llm.schema import CONTENT, ROLE, USER, Message
from qwen_agent.gui.utils import convert_history_to_chatbot
from qwen_agent.gui.gradio import gr, mgr
class OpenVINOUI(WebUI):
def request_cancel(self):
self.agent_list[0].llm.ov_model.request.cancel()
def clear_history(self):
return []
def add_text(self, _input, _chatbot, _history):
_history.append(
{
ROLE: USER,
CONTENT: [{"text": _input}],
}
)
_chatbot.append([_input, None])
yield gr.update(interactive=False, value=None), _chatbot, _history
def run(
self,
messages: List[Message] = None,
share: bool = False,
server_name: str = None,
server_port: int = None,
**kwargs,
):
self.run_kwargs = kwargs
with gr.Blocks(
theme=gr.themes.Soft(),
css=".disclaimer {font-variant-caps: all-small-caps;}",
) as self.demo:
gr.Markdown("""<h1><center>OpenVINO Qwen Agent </center></h1>""")
history = gr.State([])
with gr.Row():
with gr.Column(scale=4):
chatbot = mgr.Chatbot(
value=convert_history_to_chatbot(messages=messages),
avatar_images=[
self.user_config,
self.agent_config_list,
],
height=900,
avatar_image_width=80,
flushing=False,
show_copy_button=True,
)
with gr.Column():
input = gr.Textbox(
label="Chat Message Box",
placeholder="Chat Message Box",
show_label=False,
container=False,
)
with gr.Column():
with gr.Row():
submit = gr.Button("Submit", variant="primary")
stop = gr.Button("Stop")
clear = gr.Button("Clear")
with gr.Column(scale=1):
agent_interactive = self.agent_list[0]
capabilities = [key for key in agent_interactive.function_map.keys()]
gr.CheckboxGroup(
label="Tools",
value=capabilities,
choices=capabilities,
interactive=False,
)
with gr.Row():
gr.Examples(self.prompt_suggestions, inputs=[input], label="Click on any example and press the 'Submit' button")
input_promise = submit.click(
fn=self.add_text,
inputs=[input, chatbot, history],
outputs=[input, chatbot, history],
queue=False,
)
input_promise = input_promise.then(
self.agent_run,
[chatbot, history],
[chatbot, history],
)
input_promise.then(self.flushed, None, [input])
stop.click(
fn=self.request_cancel,
inputs=None,
outputs=None,
cancels=[input_promise],
queue=False,
)
clear.click(lambda: None, None, chatbot, queue=False).then(self.clear_history, None, history)
self.demo.load(None)
self.demo.launch(share=share, server_name=server_name, server_port=server_port)
chatbot_config = {
"prompt.suggestions": [
"Based on current weather in London, show me a picture of Big Ben",
"What is OpenVINO ?",
"Create an image of pink cat",
"What is the weather like in New York now ?",
"How many people live in Canada ?",
],
"agent.avatar": openvino_logo,
"input.placeholder": "Please input your request here",
}
demo = OpenVINOUI(
bot,
chatbot_config=chatbot_config,
)
# リモートで起動する場合は、server_name と server_port を指定
# demo.run(server_name='your server name', server_port='server port in int')
try:
demo.run()
except Exception:
demo.run(share=True)
# demo.demo.close()