OpenVINO を使用して LLM によるチャットボットを作成#
この Jupyter ノートブックは、ローカルへのインストール後にのみ起動できます。
急速に進化する人工知能 (AI) の世界では、チャットボットが顧客との対話を強化し、業務を合理化するための強力なツールとして企業に登場しました。大規模言語モデル (LLM) は、人間の言語を理解して生成できる人工知能システムです。ディープラーニング・アルゴリズムと大量のデータを使用して言語のニュアンスを学習し、一貫性のある適切な応答を生成します。適切なインテントベースのチャットボットは注文管理、FAQ、ポリシーに関する質問などの基本的なワンタッチの質問に答えることができますが、LLM チャットボットはより複雑なマルチタッチの質問に対処できます。LLM を使用すると、チャットボットがコンテキスト記憶を通じて、人間と同様の会話形式でサポートを提供できるようになります。言語モデルの機能を活用することで、チャットボットはますますインテリジェントになり、驚くべき正確さで人間の言語を理解して応答できるようになりました。
以前、OpenVINO と Optimum Intel を使用して命令に従うパイプラインを構築する方法について説明しました。参考までに Dolly の例を確認してください。このチュートリアルでは、チャット向けの大規模言語モデルを実行するため OpenVINO の機能を使用する方法を検討します。Hugging Face トランスフォーマー・ライブラリーの事前トレーニング済みモデルを使用します。ユーザー・エクスペリエンスを簡素化するために、Hugging Face の Optimum Intel ライブラリーを使用してモデルを OpenVINO™ IR 形式に変換し、推論パイプラインを作成します。推論パイプラインは OpenVINO 生成 API を使用して作成することもできます。その例については、OpenVINO 生成 API を使用した LLM チャットボットのノートブックを参照してください。
このチュートリアルは次のステップで構成されます:
前提条件のインストール
OpenVINO と Hugging Face Optimum の統合を使用して、パブリックソースからモデルをダウンロードして変換します。
NNCF を使用してモデルの重みを 4 ビットまたは 8 ビットのデータタイプに圧縮します
チャット推論パイプラインを作成します
チャット・パイプラインを実行します
目次:
必要条件#
必要な依存関係をインストールします
import os
os.environ["GIT_CLONE_PROTECTION_ACTIVE"] = "false"
%pip install -Uq pip
%pip uninstall -q -y optimum optimum-intel
%pip install --pre -Uq openvino openvino-tokenizers[transformers] --extra-index-url https://storage.openvinotoolkit.org/simple/wheels/nightly
%pip install -q --extra-index-url https://download.pytorch.org/whl/cpu\
"git+https://github.com/huggingface/optimum-intel.git"\
"git+https://github.com/openvinotoolkit/nncf.git"\
"torch>=2.1"\
"datasets"\
"accelerate"\
"gradio>=4.19"\
"onnx" "einops" "transformers_stream_generator" "tiktoken" "transformers>=4.40" "bitsandbytes"
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
import os
from pathlib import Path
import requests
import shutil
# モデル設定を取得
config_shared_path = Path("../../utils/llm_config.py")
config_dst_path = Path("llm_config.py")
if not config_dst_path.exists():
if config_shared_path.exists():
try:
os.symlink(config_shared_path, config_dst_path)
except Exception:
shutil.copy(config_shared_path, config_dst_path)
else:
r = requests.get(url="https://raw.githubusercontent.com/openvinotoolkit/openvino_notebooks/latest/utils/llm_config.py")
with open("llm_config.py", "w", encoding="utf-8") as f:
f.write(r.text)
elif not os.path.islink(config_dst_path):
print("LLM config will be updated")
if config_shared_path.exists():
shutil.copy(config_shared_path, config_dst_path)
else:
r = requests.get(url="https://raw.githubusercontent.com/openvinotoolkit/openvino_notebooks/latest/utils/llm_config.py")
with open("llm_config.py", "w", encoding="utf-8") as f:
f.write(r.text)
推論用のモデルを選択#
このチュートリアルではさまざまなモデルがサポートされており、提供されたオプションから 1 つを選択して、オープンソース LLM ソリューションの品質を比較できます。>注: 一部のモデルの変換には、ユーザーによる追加アクションが必要になる場合があり、変換には少なくとも 64GB RAM が必要です。
利用可能なオプションには以下があります:
tiny-llama-1b-chat - これは、TinyLlama/TinyLlama-1.1B-intermediate-step-1431k-3T をベースに微調整されたチャットモデルです。TinyLlama プロジェクトは、Llama 2 と同じアーキテクチャーとトークナイザーを採用し、3 兆個のトークンで 11 億個の Llama モデルを事前トレーニングすることを目的としています。これは、TinyLlama を、Llama 上に構築された多くのオープンソース・プロジェクトにプラグインできることを意味します。さらに、TinyLlama はコンパクトで、パラメーターは 1.1B のみです。このコンパクトさにより、制限された計算量とメモリー使用量を要求する多くのアプリケーションに対応できます。モデルの詳細については、モデルカードをご覧ください
mini-cpm-2b-dpo - MiniCPM は、ModelBest 社と TsinghuaNLP によって開発されたエンドサイズ LLM であり、埋め込みを除くパラメーターはわずか 24 億個です。Direct Preference Optimization (DPO) の微調整後、MiniCPM は一般的なほとんどの 7b、13b、70b モデルよりも優れたパフォーマンスを発揮します。詳細は、model_card を参照してください。
gemma-2b-it - Gemma は、Google の軽量で最先端のオープン・モデル・ファミリーであり、Gemini モデルと同じ研究とテクノロジーから構築されています。これらは、オープンウェイト、事前トレーニング済みバリアント、および命令調整済みバリアントを備えた、テキストからテキストへのデコーダーのみの大規模言語モデルであり、英語で利用できます。Gemma モデルは、質問への回答、要約、推論など、さまざまなテキスト生成タスクに適しています。このモデルは、2B パラメーター・モデルの指示調整バージョンです。モデルの詳細については、モデルカードをご覧ください。>注: デモでモデルを実行するには、ライセンス契約に同意する必要があります。>Hugging Face Hub の登録ユーザーである必要があります。HuggingFace モデルカードにアクセスし、利用規約をよく読み、同意ボタンをクリックしてください。以下のコードを実行するには、アクセストークンを使用する必要があります。アクセストークンの詳細については、ドキュメントのこのセクションを参照してください。次のコードを使用して、ノートブック環境の Hugging Face Hub にログインできます:
## 事前トレーニング済みモデルにアクセスするには、huggingfacehub にログインします
from huggingface_hub import notebook_login, whoami
try:
whoami()
print('Authorization token already provided')
except OSError:
notebook_login()
phi3-mini-instruct - Phi-3-Mini は、高品質で推論密度の高い特性に重点を置いた合成データとフィルター処理された公開ウェブサイト・データの両方を含む Phi-3 データセットでトレーニングされた、38 億のパラメーターを持つ軽量で最先端のオープンモデルです。モデルの詳細については、モデルカード、Microsoft のブログ、トレーニング・レポートをご覧ください。
red-pajama-3b-chat - GPT-NEOX アーキテクチャーに基づいた 2.8B パラメーターの事前トレーニング済み言語モデル。これは、Togetter Computer とオープンソース AI コミュニティーのリーダーによって開発されました。このモデルは、チャット機能を強化するため OASST1 および Dolly2 データセットに基づいて微調整されています。モデルの詳細については、HuggingFace モデルカードを参照してください。
gemma-7b-it - Gemma は、Google の軽量で最先端のオープン・モデル・ファミリーであり、Gemini モデルと同じ研究とテクノロジーから構築されています。これらは、オープンウェイト、事前トレーニング済みバリアント、および命令調整済みバリアントを備えた、テキストからテキストへのデコーダーのみの大規模言語モデルであり、英語で利用できます。Gemma モデルは、質問への回答、要約、推論など、さまざまなテキスト生成タスクに適しています。このモデルは、7B パラメーター・モデルの指示調整バージョンです。モデルの詳細については、モデルカードをご覧ください。>注: デモでモデルを実行するには、ライセンス契約に同意する必要があります。>Hugging Face Hub の登録ユーザーである必要があります。HuggingFace モデルカードにアクセスし、利用規約をよく読み、同意ボタンをクリックしてください。以下のコードを実行するには、アクセストークンを使用する必要があります。アクセストークンの詳細については、ドキュメントのこのセクションを参照してください。次のコードを使用して、ノートブック環境の Hugging Face Hub にログインできます:
## 事前トレーニング済みモデルにアクセスするには、huggingfacehub にログインします
from huggingface_hub import notebook_login, whoami
try:
whoami()
print('Authorization token already provided')
except OSError:
notebook_login()
llama-2-7b-chat - LLama 2 は、Meta によって開発された LLama モデルの第 2 世代です。Llama 2 は、事前トレーニングされ、微調整された生成テキストモデルのコレクションであり、その規模は 70 億から 700 億のパラメーターに及びます。 llama-2-7b-chat は、対話の使用例向けに微調整および最適化された LLama 2 の 70 億パラメーターのバージョンです。モデルの詳細については、論文、リポジトリーおよびHuggingFace モデルカード を参照してください>注: デモでモデルを実行するには、ライセンス契約に同意する必要があります。>Hugging Face Hub の登録ユーザーである必要があります。HuggingFace モデルカードにアクセスし、利用規約をよく読み、同意ボタンをクリックしてください。以下のコードを実行するには、アクセストークンを使用する必要があります。アクセストークンの詳細については、ドキュメントのこのセクションを参照してください。次のコードを使用して、ノートブック環境の Hugging Face Hub にログインできます:
## 事前トレーニング済みモデルにアクセスするには、huggingfacehub にログインします
from huggingface_hub import notebook_login, whoami
try:
whoami()
print('Authorization token already provided')
except OSError:
notebook_login()
llama-3-8b-instruct - Llama 3 は、最適化されたトランスフォーマー・アーキテクチャーを使用する自己回帰言語モデルです。調整されたバージョンでは、教師あり微調整 (SFT) と人間によるフィードバックによる強化学習 (RLHF) を使用して、有用性と安全性に関する嗜好に合わせます。Llama 3 指示調整モデルは、対話ユースケース向けに最適化されており、一般的な業界ベンチマークにおいて、多くのオープンソース・チャット・モデルよりも優れたパフォーマンスを発揮します。モデルの詳細については、Meta のブログ投稿、モデルのウェブサイトおよびモデルカード を参照してください>注: デモでモデルを実行するには、ライセンス契約に同意する必要があります。>Hugging Face Hub の登録ユーザーである必要があります。HuggingFace モデルカードにアクセスし、利用規約をよく読み、同意ボタンをクリックしてください。以下のコードを実行するには、アクセストークンを使用する必要があります。アクセストークンの詳細については、ドキュメントのこのセクションを参照してください。次のコードを使用して、ノートブック環境の Hugging Face Hub にログインできます:
## 事前トレーニング済みモデルにアクセスするには、huggingfacehub にログインします
from huggingface_hub import notebook_login, whoami
try:
whoami()
print('Authorization token already provided')
except OSError:
notebook_login()
qwen2-1.5b-instruct/qwen2-7b-instruct - Qwen2 は、Qwen 大規模言語モデルの新しいシリーズです。以前リリースされた Qwen1.5 を含む最先端のオープンソース言語モデルと比較すると、Qwen2 は一般的にほとんどのオープンソース・モデルを上回り、言語理解、言語生成、多言語機能、コーディング、数学、推論などを対象とする一連のベンチマークで独自のモデルに対して競争力があることが実証されています。詳細については、model_card、ブログ、GitHub、およびドキュメントを参照してください。
qwen1.5-0.5b-chat/qwen1.5-1.8b-chat/qwen1.5-7b-chat - Qwen1.5 は、大量のデータで事前トレーニングされたトランスフォーマー・ベースのデコーダーのみの言語モデルである Qwen2 のベータ版です。Qwen1.5 は、さまざまなモデルサイズのデコーダー言語モデルを含む言語モデルシリーズです。これは、SwiGLU 活性化、アテンション QKV バイアス、グループ・クエリー・アテンション、スライディング・ウィンドウ・アテンションとフル・アテンションの混合を備えた Transformer アーキテクチャーに基づいています。モデルの詳細については、モデルのリポジトリーを参照してください
qwen-7b-chat - Qwen-7B は、Alibaba Cloud が提案する大規模言語モデルシリーズ Qwen (略称 Tongyi Qianwen) の 7B パラメーター・バージョンです。Qwen-7B は、ウェブテキスト、書籍、コードなどを含む大量のデータで事前トレーニングされた Transformer ベースの大規模言語モデルです。Qwen の詳細については、GitHub コード・リポジトリーを参照してください。
mpt-7b-chat - MPT-7B は MosaicPretrainedTransformer (MPT) モデルファミリーの一部であり、効率的なトレーニングと推論のために最適化された修正されたトランスフォーマー・アーキテクチャーを使用します。これらのアーキテクチャー変更には、パフォーマンスが最適化されたレイヤーの実装と、位置埋め込みを線形バイアスによるアテンション (ALiBi) に置き換えることによるコンテキストの長さの制限の排除が含まれます。これらの変更により、MPT モデルは高いスループット効率と安定した収束でトレーニングできます。MPT-7B-chat は、対話生成のためのチャットボットのようなモデルです。これは、ShareGPT-Vicuna、HC3、Alpaca、HH-RLHF、および Evol-Instruct データセット上で MPT-7B を微調整することによって構築されました。モデルの詳細については、ブログのポスト、リポジトリーおよびHuggingFace モデルカード を参照してください
chatglm3-6b - ChatGLM3-6B は、ChatGLM シリーズの最新のオープンソース・モデルです。hatGLM3-6B は、前の 2 世代のスムーズな対話や低い導入しきい値など多くの優れた機能を維持しながら、多様なトレーニング・データセット、十分なトレーニング・ステップ、合理的なトレーニング戦略を採用しています。ChatGLM3-6B では、通常のマルチターン・ダイアログに加え、新たに設計されたプロンプト形式を採用しています。モデルの詳細については、モデルカードを参照してください
mistral-7b - Mistral-7B-v0.1 大規模言語モデル (LLM) は、70 億のパラメーターを備えた事前トレーニング済みの生成テキストモデルです。モデルの詳細については、モデルカード、論文およびリリースのブログポストを参照してください。
zephyr-7b-beta - Zephyr は、有用なアシスタントとして機能するように訓練された一連の言語モデルです。Zephyr-7B-beta はシリーズの 2 番目のモデルであり、直接優先最適化 (DPO) を使用して公開されている合成データセットの組み合わせでトレーニングされた、mistralai/Mistral-7B-v0.1 の微調整されたバージョンです。モデルの詳細については、技術レポートおよび HuggingFace モデルカードを参照してください。
neural-chat-7b-v3-1 - インテル® Gaudi を使用して微調整された Mistral-7b モデル。モデルはオープンソース・データセット Open-Orca/SlimOrca に基づいて微調整され、直接優先最適化 (DPO) アルゴリズムに合わせて調整されています。詳細については、モデルカードとブログの投稿をご覧ください。
notus-7b-v1 - Notus は、直接優先最適化 (DPO) および関連する RLHF 技術を使用して微調整されたモデルのコレクションです。このモデルは、zephyr-7b-sft を DPO で微調整した最初のバージョンです。データファーストのアプローチに従って、Notus-7B-v1 と Zephyr-7B-beta の唯一の違いは、dDPO に使用される優先データセットです。データセットの作成に提案されたアプローチは、AlpacaEval 上の Zephyr-7B-beta や Claude 2 を超える Notus-7b を効果的に微調整するのに役立ちます。モデルの詳細については、モデルカードをご覧ください。
youri-7b-chat - Youri-7b-chat は Llama2 ベースのモデルです。Rinna Co., Ltd. は、日本語のタスク能力を向上させるために、英語と日本語のデータセットを混合した Llama2 モデルの事前トレーニングをさらに行いました。モデルは Hugging Face ハブで公開されています。詳しい情報は rinna/youri-7b-chat プロジェクトのページでご覧いただけます。
baichuan2-7b-chat - Baichuan 2 は、Baichuan Intelligence 社が発表した新世代の大規模オープンソース言語モデルです。2.6 兆トークンの高品質コーパスでトレーニングされており、同じサイズの権威ある中国語と英語のベンチマークで最高のパフォーマンスを達成しています。
internlm2-chat-1.8b - InternLM2 は、第 2 世代の InternLM シリーズです。前世代のモデルと比較すると、推論、数学、コーディングなど、さまざまな機能が大幅に向上しています。モデルの詳細については、モデル・リポジトリーをご覧ください。
glm-4-9b-chat - GLM-4-9B は、Zhipu AI が発売した GLM-4 シリーズの最新世代の事前トレーニング済みモデルのオープンソース・バージョンです。セマンティクス、数学、推論、コード、知識のデータセットの評価では、GLM-4-9B とその人間の好みに合わせたバージョンである GLM-4-9B-Chat は、Llama-3-8B よりも優れたパフォーマンスを示しました。GLM-4-9B-Chat には、複数ラウンドの会話に加えて、ウェブ・ブラウジング、コード実行、カスタムツール呼び出し (関数呼び出し)、長文テキストの推論 (最大 128K のコンテキストをサポート) などの高度な機能もあります。モデルの詳細については、モデルカード、技術レポート、およびリポジトリーをご覧ください。
from llm_config import SUPPORTED_LLM_MODELS
import ipywidgets as widgets
model_languages = list(SUPPORTED_LLM_MODELS)
model_language = widgets.Dropdown(
options=model_languages,
value=model_languages[0],
description="Model Language:",
disabled=False,
)
model_language
Dropdown(description='Model Language:', options=('English', 'Chinese', 'Japanese'), value='English')
model_ids = list(SUPPORTED_LLM_MODELS[model_language.value])
model_id = widgets.Dropdown(
options=model_ids,
value=model_ids[0],
description="Model:",
disabled=False,
)
model_id
Dropdown(description='Model:', index=2, options=('tiny-llama-1b-chat', 'gemma-2b-it', 'phi-3-mini-instruct', '…
model_configuration = SUPPORTED_LLM_MODELS[model_language.value][model_id.value]
print(f"Selected model {model_id.value}")
Selected model qwen2-7b-instruct
Optimum-CLI ツールを使用してモデルを変換#
Optimum Intel は、インテル® アーキテクチャー上のエンドツーエンドのパイプラインを高速化する、トランスフォーマーおよび Diffuser ライブラリーと OpenVINO 間のインターフェイスです。モデルを OpenVINO 中間表現 (IR) 形式にエクスポートする使いやすい CLI インターフェイスを提供します。
以下は、optimum-cli
を使用したモデル・エクスポートの基本コマンドを示しています。
optimum-cli export openvino --model <model_id_or_path> --task <task> <out_dir>
--model
l 引数は、HuggingFace Hub またはモデルのあるローカル・ディレクトリー (.save_pretrained
メソッドを使用して保存) のモデル ID であり、--task
は、エクスポートされたモデルが解決する必要があるサポートされているタスクの 1 つです。LLM の場合は、text-generation-with-past
になります。モデルの初期化にリモートコードを使用する場合は、--trust-remote-code
フラグを渡す必要があります。
モデルの重みを圧縮#
重み圧縮アルゴリズムは、モデルの重みを圧縮することを目的としており、大規模言語モデル (LLM) など、重みのサイズが活性化のサイズよりも相対的に大きい大規模モデルのモデル・フットプリントとパフォーマンスを最適化するために使用できます。INT8 圧縮と比較して、INT4 圧縮はパフォーマンスをさらに向上させますが、予測品質は若干低下します。
Optimum-CLI を使用した重み圧縮#
また、CLI を使用してモデルをエクスポートするときに、--weight-format をそれぞれ fp16、int8、int4 に設定することで、線形、畳み込み、埋め込みレイヤーに fp16、8 ビット、または 4 ビットの重み圧縮を適用することもできます。このタイプ最適化により、メモリー・フットプリントと推論の待ち時間を削減できます。デフォルトでは、int8/int4 の量子化スキームは非対称になりますが、対称にするには --sym を追加します。
INT4 量子化の場合、次の引数を指定することもできます:
--group-size
パラメーターは量子化に使用するグループサイズを定義します。-1 の場合はカラムごとの量子化になります。--ratio
パラメーターは、4 ビットと 8 ビットの量子化の比率を制御します。例えば、0.8 は、レイヤーの 90% が int4 に圧縮され、残りが int8 精度に圧縮されることを意味します。
group_size と ratio の値が小さいほど、モデルのサイズと推論のレイテンシーが犠牲になりますが、通常は精度が向上します。
注: dGPU 上の INT4/INT8 圧縮モデルではスピードアップされない可能性があります。
from IPython.display import Markdown, display
prepare_int4_model = widgets.Checkbox(
value=True,
description="Prepare INT4 model",
disabled=False,
)
prepare_int8_model = widgets.Checkbox(
value=False,
description="Prepare INT8 model",
disabled=False,
)
prepare_fp16_model = widgets.Checkbox(
value=False,
description="Prepare FP16 model",
disabled=False,
)
display(prepare_int4_model)
display(prepare_int8_model)
display(prepare_fp16_model)
Checkbox(value=True, description='Prepare INT4 model')
Checkbox(value=False, description='Prepare INT8 model')
Checkbox(value=False, description='Prepare FP16 model')
AWQ を使用した重み圧縮#
活性化認識重み量子化 (AWQ) は、モデルの重みを調整して INT4 圧縮の精度を高めるアルゴリズムです。圧縮された LLM の生成品質はわずかに向上しますが、キャリブレーション・データセットの重みを調整するのにかなりの時間が必要になります。キャリブレーションには、Wikitext データセットの wikitext-2-raw-v1/train
サブセットを使用します。
以下では、INT4 精度でモデルのエクスポート中に AWQ を適用できるようにします。
注: AWQ を適用するには、かなりのメモリーと時間が必要です。
注: AWQ を適用するモデルに一致するパターンが存在しない可能性があり、その場合はスキップされます。
enable_awq = widgets.Checkbox(
value=False,
description="Enable AWQ",
disabled=not prepare_int4_model.value,
)
display(enable_awq)
浮動小数点と圧縮モデルのバリアントを保存できるようになりました
from pathlib import Path
pt_model_id = model_configuration["model_id"]
pt_model_name = model_id.value.split("-")[0]
fp16_model_dir = Path(model_id.value) / "FP16"
int8_model_dir = Path(model_id.value) / "INT8_compressed_weights"
int4_model_dir = Path(model_id.value) / "INT4_compressed_weights"
def convert_to_fp16():
if (fp16_model_dir / "openvino_model.xml").exists():
return
remote_code = model_configuration.get("remote_code", False)
export_command_base = "optimum-cli export openvino --model {} --task text-generation-with-past --weight-format fp16".format(pt_model_id)
if remote_code:
export_command_base += " --trust-remote-code"
export_command = export_command_base + " " + str(fp16_model_dir)
display(Markdown("**Export command:**"))
display(Markdown(f"`{export_command}`"))
! $export_command
def convert_to_int8():
if (int8_model_dir / "openvino_model.xml").exists():
return
int8_model_dir.mkdir(parents=True, exist_ok=True)
remote_code = model_configuration.get("remote_code", False)
export_command_base = "optimum-cli export openvino --model {} --task text-generation-with-past --weight-format int8".format(pt_model_id)
if remote_code:
export_command_base += " --trust-remote-code"
export_command = export_command_base + " " + str(int8_model_dir)
display(Markdown("**Export command:**"))
display(Markdown(f"`{export_command}`"))
! $export_command
def convert_to_int4():
compression_configs = {
"zephyr-7b-beta": { "sym": True,
"group_size": 64,
"ratio": 0.6,
},
"mistral-7b": {
"sym": True,
"group_size": 64,
"ratio": 0.6,
},
"minicpm-2b-dpo": {
"sym": True,
"group_size": 64,
"ratio": 0.6,
},
"gemma-2b-it": {
"sym": True,
"group_size": 64,
"ratio": 0.6,
},
"notus-7b-v1": {
"sym": True,
"group_size": 64,
"ratio": 0.6,
},
"neural-chat-7b-v3-1": {
"sym": True,
"group_size": 64,
"ratio": 0.6,
},
"llama-2-chat-7b": {
"sym": True,
"group_size": 128,
"ratio": 0.8,
},
"llama-3-8b-instruct": {
"sym": True,
"group_size": 128,
"ratio": 0.8,
},
"gemma-7b-it": {
"sym": True,
"group_size": 128,
"ratio": 0.8,
},
"chatglm2-6b": {
"sym": True,
"group_size": 128,
"ratio": 0.72,
},
"qwen-7b-chat": {"sym": True, "group_size": 128, "ratio": 0.6},
"red-pajama-3b-chat": {
"sym": False,
"group_size": 128,
"ratio": 0.5,
},
"default": {
"sym": False,
"group_size": 128,
"ratio": 0.8,
},
}
model_compression_params = compression_configs.get(model_id.value, compression_configs["default"])
if (int4_model_dir / "openvino_model.xml").exists():
return
remote_code = model_configuration.get("remote_code", False)
export_command_base = "optimum-cli export openvino --model {} --task text-generation-with-past --weight-format int4".format(pt_model_id)
int4_compression_args = " --group-size {} --ratio {}".format(model_compression_params["group_size"], model_compression_params["ratio"])
if model_compression_params["sym"]:
int4_compression_args += " --sym"
if enable_awq.value:
int4_compression_args += " --awq --dataset wikitext2 --num-samples 128"
export_command_base += int4_compression_args
if remote_code:
export_command_base += " --trust-remote-code"
export_command = export_command_base + " " + str(int4_model_dir)
display(Markdown("**Export command:**"))
display(Markdown(f"`{export_command}`"))
! $export_command
if prepare_fp16_model.value:
convert_to_fp16()
if prepare_int8_model.value:
convert_to_int8()
if prepare_int4_model.value:
convert_to_int4()
エクスポート・コマンド:
optimum-cli export openvino --model Qwen/Qwen2-7B-Instruct --task text-generation-with-past --weight-format int4 --group-size 128 --ratio 0.8 qwen2-7b-instruct/INT4_compressed_weights
さまざまな圧縮タイプのモデルサイズを比較してみましょう
fp16_weights = fp16_model_dir / "openvino_model.bin"
int8_weights = int8_model_dir / "openvino_model.bin"
int4_weights = int4_model_dir / "openvino_model.bin"
if fp16_weights.exists():
print(f"Size of FP16 model is {fp16_weights.stat().st_size / 1024 / 1024:.2f} MB")
for precision, compressed_weights in zip([8, 4], [int8_weights, int4_weights]):
if compressed_weights.exists():
print(f"Size of model with INT{precision} compressed weights is {compressed_weights.stat().st_size / 1024 / 1024:.2f} MB")
if compressed_weights.exists() and fp16_weights.exists():
print(f"Compression rate for INT{precision} model: {fp16_weights.stat().st_size / compressed_weights.stat().st_size:.3f}")
Size of model with INT4 compressed weights is 4929.13 MB
推論用のデバイスとモデルバリアントを選択#
注: dGPU 上の INT4/INT8 圧縮モデルではスピードアップされない可能性があります。
import openvino as ov
core = ov.Core()
support_devices = core.available_devices
if "NPU" in support_devices:
support_devices.remove("NPU")
device = widgets.Dropdown(
options=support_devices + ["AUTO"],
value="CPU",
description="Device:",
disabled=False,
)
device
Dropdown(description='Device:', options=('CPU', 'GPU.0', 'GPU.1', 'AUTO'), value='CPU')
以下のセルは、モデルの重みと推論デバイスの選択されたバリアントに基づいてモデルをインスタンス化する方法を示しています
available_models = []
if int4_model_dir.exists():
available_models.append("INT4")
if int8_model_dir.exists():
available_models.append("INT8")
if fp16_model_dir.exists():
available_models.append("FP16")
model_to_run = widgets.Dropdown(
options=available_models,
value=available_models[0],
description="Model to run:",
disabled=False,
)
model_to_run
Dropdown(description='Model to run:', options=('INT4',), value='INT4')
Optimum Intel を使用してモデルをインスタンス化#
Optimum Intel を使用すると、Hugging Face ハブ から最適化されたモデルをロードし、Hugging Face API を使用して OpenVINO ランタイムで推論を実行するパイプラインを作成できます。Optimum 推論モデルは、Hugging Face Transformers モデルと API の互換性があります。つまり、AutoModelForXxx
クラスを対応する OVModelForXxx
クラスに置き換えるだけで済みます。
以下は RedPajama モデルの例です
-from transformers import AutoModelForCausalLM
+from optimum.intel.openvino import OVModelForCausalLM
from transformers import AutoTokenizer, pipeline
model_id = "togethercomputer/RedPajama-INCITE-Chat-3B-v1"
-model = AutoModelForCausalLM.from_pretrained(model_id)
+model = OVModelForCausalLM.from_pretrained(model_id, export=True)
モデルクラスの初期化は、from_pretrained
メソッドの呼び出しから始まります。トランスフォーマー・モデルをダウンロードして変換する場合、export=True
パラメーターを追加する必要がありますが、以前にモデルを変換したので、このパラメーターを指定する必要はありません。save_pretrained
メソッドを使用して、変換されたモデルを次回に使用するため保存できます。トークナイザー・クラスとパイプライン API は Optimum モデルと互換性があります。
HuggingFace Optimum API を使用した OpenVINO LLM 推論の詳細については、LLM 推論ガイドを参照してください。
from transformers import AutoConfig, AutoTokenizer
from optimum.intel.openvino import OVModelForCausalLM
if model_to_run.value == "INT4":
model_dir = int4_model_dir
elif model_to_run.value == "INT8":
model_dir = int8_model_dir
else:
model_dir = fp16_model_dir
print(f"Loading model from {model_dir}")
ov_config = {"PERFORMANCE_HINT": "LATENCY", "NUM_STREAMS": "1", "CACHE_DIR": ""}
if "GPU" in device.value and "qwen2-7b-instruct" in model_id.value:
ov_config["GPU_ENABLE_SDPA_OPTIMIZATION"] = "NO"
# GPU デバイスでは、モデルは FP16 精度で実行されます。red-pajama-3b-chat モデルでは、これが原因で精度の問題が発生することがありますが、
# 精度ヒントを "f32" に設定することでこれを回避できます
if model_id.value == "red-pajama-3b-chat" and "GPU" in core.available_devices and device.value in ["GPU", "AUTO"]:
ov_config["INFERENCE_PRECISION_HINT"] = "f32"
model_name = model_configuration["model_id"]
tok = AutoTokenizer.from_pretrained(model_dir, trust_remote_code=True)
ov_model = OVModelForCausalLM.from_pretrained(
model_dir,
device=device.value,
ov_config=ov_config,
config=AutoConfig.from_pretrained(model_dir, trust_remote_code=True),
trust_remote_code=True,
)
Loading model from qwen2-7b-instruct/INT4_compressed_weights
Special tokens have been added in the vocabulary, make sure the associated word embeddings are fine-tuned or trained. The argument trust_remote_code is to be used along with export=True. It will be ignored.Compiling the model to CPU ...
tokenizer_kwargs = model_configuration.get("tokenizer_kwargs", {})
test_string = "2 + 2 ="
input_tokens = tok(test_string, return_tensors="pt", **tokenizer_kwargs)
answer = ov_model.generate(**input_tokens, max_new_tokens=2)
print(tok.batch_decode(answer, skip_special_tokens=True)[0])
2 + 2 = 4
チャットボットを実行#
モデルが作成されたら、Gradio を使用してチャットボット・インターフェイスをセットアップできます。以下の図は、チャットボット・パイプラインがどのように機能するかを示しています
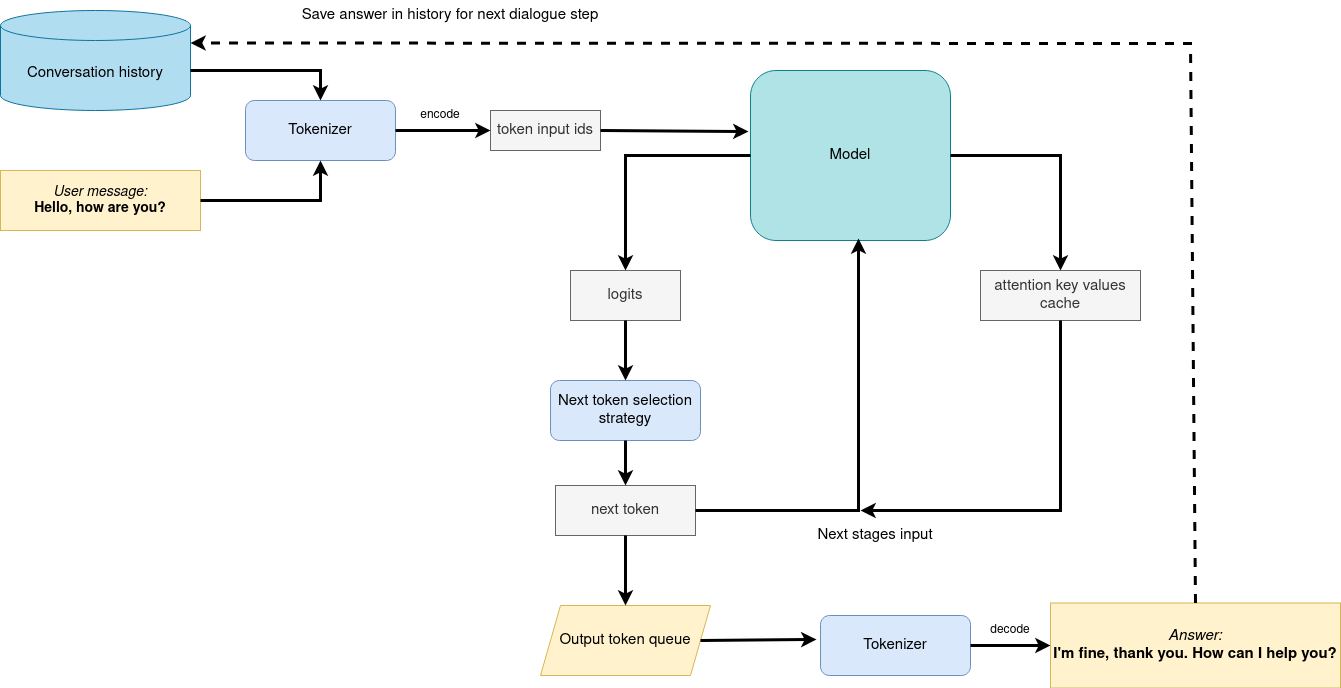
生成パイプライン#
パイプラインは命令フォローに非常によく似ていますが、より幅広い入力コンテキストを取得するために、以前の会話履歴が次のユーザーの質問で入力としてさらに渡されることが変更されただけです。最初の反復では、ユーザーが提供した指示が会話履歴 (存在する場合) に結合され、トークナイザーを使用してトークン ID に変換され、モデルに提供される準備入力が行われました。モデルは、すべてのトークンの確率をロジット形式で生成します。予測された確率に基づいて次のトークンが選択される方法は、選択されたデコード方法によって決まります。最も一般的なデコード方法の詳細については、このブログをご覧ください。結果の生成により、次の会話ステップの会話履歴が更新されます。これにより、次の質問と以前の質問との結びつきがより強くなり、以前の回答について説明できるようになります: https://docs.openvino.ai/2024/learn-openvino/llm_inference_guide.html
Temperature
は、AI が生成したテキストの創造性のレベルを制御するパラメーターです。temperature
を調整することで、AI モデルの確率分布に影響を与え、テキストの焦点を絞ったり、多様にしたりできます。playing: 0.5
sleeping: 0.25
eating: 0.15
driving: 0.05
flying: 0.05
- **Low temperature** (e.g., 0.2): The AI model becomes more focused and deterministic, choosing tokens with the highest probability, such as "playing."
- **Medium temperature** (e.g., 1.0): The AI model maintains a balance between creativity and focus, selecting tokens based on their probabilities without significant bias, such as "playing," "sleeping," or "eating."
- **High temperature** (e.g., 2.0): The AI model becomes more adventurous, increasing the chances of selecting less likely tokens, such as "driving" and "flying."
Top-p
は、核サンプリングとも呼ばれる累積確率に基づいて AI モデルによって考慮される、トークンの範囲を制御するパラメーターです。top-p
値を調整することで、AI モデルのトークン選択に影響を与え、焦点を絞ったり、多様性を持たせることができます。猫と同じ例を使用して、次の top_p 設定を検討してください:低 top_p (例 0.5): AI モデルは、 “playing” など、累積確率が最も高いトークンのみを考慮します。
中 top_p (例 0.8): AI モデルは、“playing”、“sleeping”、“eating” など、累積確率がより高いトークンを考慮します。
高 top_p (例 1.0): AI モデルは、“driving” や “flying” などの確率の低いトークンを含むすべてのトークンを考慮します。
Top-k
は、人気のあるサンプリング戦略です。累積確率が確率 P を超える最小の単語のセットから選択する Top-P と比較して、Top-K サンプリングでは、次の可能性が最も高い K 個の単語がフィルタリングされ、確率の集合が K 個の次の単語のみに再分配されます。猫の例では、k=3 の場合、“playing”、“sleeping” および “eating” だけが次の単語として考慮されます。Repetition Penalty
このパラメーターは、入力プロンプトを含むテキスト内でのトークンの出現頻度に基づいてトークンにペナルティーを与えるのに役立ちます。5 回出現したトークンは、1 回しか出現しなかったトークンよりも重いペナルティーが課されます。値 1 はペナルティーがないことを意味し、値が 1 より大きい場合、トークンの反復が妨げられます: https://docs.openvino.ai/2024/learn-openvino/llm_inference_guide.html
import torch
from threading import Event, Thread
from uuid import uuid4
from typing import List, Tuple
import gradio as gr
from transformers import (
AutoTokenizer,
StoppingCriteria,
StoppingCriteriaList,
TextIteratorStreamer,
)
model_name = model_configuration["model_id"]
start_message = model_configuration["start_message"]
history_template = model_configuration.get("history_template")
current_message_template = model_configuration.get("current_message_template")
stop_tokens = model_configuration.get("stop_tokens")
tokenizer_kwargs = model_configuration.get("tokenizer_kwargs", {})
chinese_examples = [
["你好!"],
["你是谁?"],
["请介绍一下上海"],
["请介绍一下英特尔公司"],
["晚上睡不着怎么办?"],
["给我讲一个年轻人奋斗创业最终取得成功的故事。"],
["给这个故事起一个标题。"],
]
english_examples = [
["Hello there! How are you doing?"],
["What is OpenVINO?"],
["Who are you?"],
["Can you explain to me briefly what is Python programming language?"],
["Explain the plot of Cinderella in a sentence."],
["What are some common mistakes to avoid when writing code?"],
["Write a 100-word blog post on “Benefits of Artificial Intelligence and OpenVINO“"],
]
japanese_examples = [
["こんにちは!調子はどうですか?"],
["OpenVINO とは何ですか?"],
["あなたは誰ですか?"],
["Python プログラミング言語とは何か簡単に説明してもらえますか?"],
["シンデレラのあらすじを一文で説明してください。"],
["コードを書くときに避けるべきよくある間違いは何ですか?"],
["人工知能と「OpenVINO の利点」について 100 語程度のブログ記事を書いてください。"],
]
examples = chinese_examples if (model_language.value == "Chinese") else japanese_examples if (model_language.value == "Japanese") else english_examples
max_new_tokens = 256
class StopOnTokens(StoppingCriteria):
def __init__(self, token_ids):
self.token_ids = token_ids
def __call__(self, input_ids: torch.LongTensor, scores: torch.FloatTensor, **kwargs) -> bool:
for stop_id in self.token_ids:
if input_ids[0][-1] == stop_id:
return True
return False
if stop_tokens is not None:
if isinstance(stop_tokens[0], str):
stop_tokens = tok.convert_tokens_to_ids(stop_tokens)
stop_tokens = [StopOnTokens(stop_tokens)]
def default_partial_text_processor(partial_text: str, new_text: str):
"""
helper for updating partially generated answer, used by default
Params:
partial_text: text buffer for storing previosly generated text
new_text: text update for the current step
Returns:
updated text string
"""
partial_text += new_text
return partial_text
text_processor = model_configuration.get("partial_text_processor", default_partial_text_processor)
def convert_history_to_token(history: List[Tuple[str, str]]):
"""
function for conversion history stored as list pairs of user and assistant messages to tokens according to model expected conversation template
Params:
history: dialogue history
Returns:
history in token format
"""
if pt_model_name == "baichuan2":
system_tokens = tok.encode(start_message)
history_tokens = []
for old_query, response in history[:-1]:
round_tokens = []
round_tokens.append(195)
round_tokens.extend(tok.encode(old_query))
round_tokens.append(196)
round_tokens.extend(tok.encode(response))
history_tokens = round_tokens + history_tokens input_tokens = system_tokens + history_tokens
input_tokens.append(195)
input_tokens.extend(tok.encode(history[-1][0]))
input_tokens.append(196)
input_token = torch.LongTensor([input_tokens])
elif history_template is None:
messages = [{"role": "system", "content": start_message}]
for idx, (user_msg, model_msg) in enumerate(history):
if idx == len(history) - 1 and not model_msg:
messages.append({"role": "user", "content": user_msg})
break
if user_msg:
messages.append({"role": "user", "content": user_msg})
if model_msg:
messages.append({"role": "assistant", "content": model_msg})
input_token = tok.apply_chat_template(messages, add_generation_prompt=True, tokenize=True, return_tensors="pt")
else:
text = start_message + "".join(
["".join([history_template.format(num=round, user=item[0], assistant=item[1])]) for round, item in enumerate(history[:-1])]
)
text += "".join(
[
"".join(
[
current_message_template.format(
num=len(history) + 1,
user=history[-1][0],
assistant=history[-1][1],
)
]
)
]
)
input_token = tok(text, return_tensors="pt", **tokenizer_kwargs).input_ids
return input_token
def user(message, history):
"""
callback function for updating user messages in interface on submit button click
Params:
message: current message
history: conversation history
Returns:
None
"""
# ユーザーのメッセージを会話履歴に追加
return "", history + [[message, ""]]
def bot(history, temperature, top_p, top_k, repetition_penalty, conversation_id):
"""
callback function for running chatbot on submit button click
Params:
history: conversation history
temperature: parameter for control the level of creativity in AI-generated text.
By adjusting the `temperature`, you can influence the AI model's probability distribution, making the text more focused or diverse.
top_p: parameter for control the range of tokens considered by the AI model based on their cumulative probability.
top_k: parameter for control the range of tokens considered by the AI model based on their cumulative probability, selecting number of tokens with highest probability.
repetition_penalty: parameter for penalizing tokens based on how frequently they occur in the text.
conversation_id: unique conversation identifier.
"""
# 現在のシステムメッセージと会話履歴を連結して、モデルの入力メッセージ文字列を構築
# メッセージの文字列をトークン化
input_ids = convert_history_to_token(history)
if input_ids.shape[1] > 2000:
history = [history[-1]]
input_ids = convert_history_to_token(history)
streamer = TextIteratorStreamer(tok, timeout=30.0, skip_prompt=True, skip_special_tokens=True)
generate_kwargs = dict(
input_ids=input_ids,
max_new_tokens=max_new_tokens,
temperature=temperature,
do_sample=temperature > 0.0,
top_p=top_p,
top_k=top_k,
repetition_penalty=repetition_penalty,
streamer=streamer,
)
if stop_tokens is not None:
generate_kwargs["stopping_criteria"] = StoppingCriteriaList(stop_tokens)
stream_complete = Event()
def generate_and_signal_complete():
"""
genration function for single thread
"""
global start_time
ov_model.generate(**generate_kwargs)
stream_complete.set()
t1 = Thread(target=generate_and_signal_complete)
t1.start()
# 生成されたテキストを保存するため空の文字列を初期化
partial_text = ""
for new_text in streamer:
partial_text = text_processor(partial_text, new_text)
history[-1][1] = partial_text
yield history
def request_cancel(): ov_model.request.cancel() def get_uuid():
"""
universal unique identifier for thread
"""
return str(uuid4())
with gr.Blocks(
theme=gr.themes.Soft(),
css=".disclaimer {font-variant-caps: all-small-caps;}",
) as demo:
conversation_id = gr.State(get_uuid)
gr.Markdown(f"""<h1><center>OpenVINO {model_id.value} Chatbot</center></h1>""")
chatbot = gr.Chatbot(height=500)
with gr.Row():
with gr.Column():
msg = gr.Textbox(
label="Chat Message Box",
placeholder="Chat Message Box",
show_label=False,
container=False,
)
with gr.Column():
with gr.Row():
submit = gr.Button("Submit")
stop = gr.Button("Stop")
clear = gr.Button("Clear")
with gr.Row():
with gr.Accordion("Advanced Options:", open=False):
with gr.Row():
with gr.Column():
with gr.Row():
temperature = gr.Slider(
label="Temperature",
value=0.1,
minimum=0.0,
maximum=1.0,
step=0.1,
interactive=True,
info="Higher values produce more diverse outputs",
)
with gr.Column():
with gr.Row():
top_p = gr.Slider(
label="Top-p (nucleus sampling)",
value=1.0,
minimum=0.0,
maximum=1,
step=0.01,
interactive=True,
info=(
"Sample from the smallest possible set of tokens whose cumulative probability "
"exceeds top_p. Set to 1 to disable and sample from all tokens." ),
)
with gr.Column():
with gr.Row():
top_k = gr.Slider(
label="Top-k",
value=50,
minimum=0.0,
maximum=200,
step=1,
interactive=True,
info="Sample from a shortlist of top-k tokens — 0 to disable and sample from all tokens.",
)
with gr.Column():
with gr.Row():
repetition_penalty = gr.Slider(
label="Repetition Penalty",
value=1.1,
minimum=1.0,
maximum=2.0,
step=0.1,
interactive=True,
info="Penalize repetition — 1.0 to disable.",
)
gr.Examples(examples, inputs=msg, label="Click on any example and press the 'Submit' button")
submit_event = msg.submit(
fn=user,
inputs=[msg, chatbot],
outputs=[msg, chatbot],
queue=False,
).then(
fn=bot,
inputs=[
chatbot,
temperature,
top_p,
top_k,
repetition_penalty,
conversation_id,
],
outputs=chatbot,
queue=True,
)
submit_click_event = submit.click(
fn=user,
inputs=[msg, chatbot],
outputs=[msg, chatbot],
queue=False,
).then(
fn=bot,
inputs=[
chatbot,
temperature,
top_p,
top_k,
repetition_penalty,
conversation_id,
],
outputs=chatbot,
queue=True,
)
stop.click(
fn=request_cancel,
inputs=None,
outputs=None,
cancels=[submit_event, submit_click_event],
queue=False,
)
clear.click(lambda: None, None, chatbot, queue=False)
# リモートで起動する場合は、server_name と server_port を指定
# demo.launch(server_name='your server name', server_port='server port in int')
# プラットフォーム上で起動する際に問題がある場合は、起動メソッドに share=True を渡すことができます:
# demo.launch(share=True)
# インターフェイスの公開共有可能なリンクを作成。詳細はドキュメントをご覧ください: https://gradio.app/docs/
demo.launch()
# gradio インターフェイスを停止するには、このセルのコメントを外して実行します
# demo.close()
次のステップ#
チャットボット以外にも、LangChain を使用して LLM 知識を追加データで拡張し、個人データやモデルのカットオフ以降に導入されたデータを推論できる AI アプリケーションを構築できます。このソリューションは、検索拡張生成 (RAG) の例で見つけることができます。