OpenVINO™ による人物追跡#
この Jupyter ノートブックはオンラインで起動でき、ブラウザーのウィンドウで対話型環境を開きます。ローカルにインストールすることもできます。次のオプションのいずれかを選択します:
このノートブックは、OpenVINO を使用したライブ人物追跡のデモンストレーションを行います。入力ビデオシーケンスからフレームを読み取り、フレーム内の人物を検出し、各人物を一意に識別して、フレームから出るまで全員を追跡します。SORT (Simple Online and Realtime Tracking) の拡張である Deep SORT アルゴリズムを使用してオブジェクト追跡を実行します。
検出と追跡#
オブジェクト検出では、フレーム内のオブジェクトを検出し、その周囲に境界ボックスまたはマスクを配置して、オブジェクトを分類します。検出器のジョブはここで終了することに注意してください。各フレームを個別に処理し、その特定のフレーム内の多数のオブジェクトを識別します。
一方、オブジェクト・トラッカーは、ビデオ全体にわたって特定のオブジェクトを追跡する必要があります。検出器がフレーム内で 3 台の車を検出した場合、オブジェクト・トラッカーは 3 つの個別の検出を識別し、後続のフレームにわたってそれを追跡する必要があります (一意の ID で)。
Deep SORT#
Deep SORT は、オブジェクトの速度と動きだけでなく、オブジェクトの外観に基づいてオブジェクトを追跡するアルゴリズムとして定義できます。これは次の 3 つの主要コンポーネントで構成されています:
検出
これは追跡モジュールの最初のステップです。このステップでは、ディープラーニング・モデルを使用して、フレーム内の追跡対象となるオブジェクトを検出します。これらの検出は次のステップに渡されます。
予測
このステップでは、カルマンフィルター[1] フレームワークを使用して、次のフレームの各追跡オブジェクトのターゲット境界ボックスを予測します。予測出力には、2 つの状態があります:
confirmed
とunconfirmed
。新しいトラックはデフォルトでunconfirmed
(未確認) の状態になっていますが、この新しいトラックと一定数の連続検出が一致すると、confirmed
(確認済み) にすることができます。一方、一致したトラックが一定時間以上再生されなかった場合、そのトラックも削除されます。データの関連付けと更新
ここで、ターゲット境界ボックスを検出された境界ボックスと一致させ、トラック ID を更新する必要があります。予測されたカルマン状態と新たに到達した測定値との関連を解決する従来の方法は、ハンガリー・アルゴリズム[2] を使用して割り当て問題を構築することです。この問題の定式化では、2 つの適切なメトリックの組み合わせを通じて、動きと外観の情報を統合します。最初のマッチングステップで使用されるコストは、マハラノビス距離とコサイン距離の組み合わせとして設定されます。マハラノビス距離は動き情報を組み込むために使用され、コサイン距離は 2 つのオブジェクト間の類似性を計算するために使用されます。コサイン距離は、長期間の遮蔽や動きの推定が失敗した場合にトラッカーが ID を回復するのに役立つメトリックです。この目的のため、再識別モデルが実装され、オブジェクトの外観を表す高次元空間のベクトルが生成されます。これら単純なものを使用することで、トラッカーはさらに強力かつ正確になります。
2 番目のマッチングステージでは、前のステップで未確認かつ一致しなかったトラックのセットに対して、オリジナルの SORT アルゴリズム[3] で提案された交差和集合 (IOU) 関連付けを実行します。検出とターゲットの IOU が
IOUmin
と呼ばれるしきい値より小さい場合、その割り当ては拒否されます。これにより、静的なシーン・ジオメトリーによる部分的な遮蔽などによる突然の外観の変化を考慮し、エラーに対する堅牢性を高めることができます。検出結果がターゲットに関連付けられると、検出された境界ボックスを使用してターゲットの状態が更新されます。
[1] R. Kalman, “A New Approach to Linear Filtering and Prediction Problems”, Journal of Basic Engineering, vol. 82, no. Series D, pp. 35-45, 1960.
[2] H. W. Kuhn, “The Hungarian method for the assignment problem”, Naval Research Logistics Quarterly, vol. 2, pp. 83-97, 1955.
[3] A. Bewley, G. Zongyuan, F. Ramos, and B. Upcroft, “Simple online and realtime tracking,” in ICIP, 2016, pp. 3464–3468.
目次:
import platform
%pip install -q "openvino-dev>=2024.0.0"
%pip install -q opencv-python requests scipy tqdm
if platform.system() != "Windows":
%pip install -q "matplotlib>=3.4"
else:
%pip install -q "matplotlib>=3.4,<3.7"
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
インポート#
import collections
from pathlib import Path
import time
import numpy as np
import cv2
from IPython import display
import matplotlib.pyplot as plt
import openvino as ov
# ローカルモジュールをインポート
if not Path("./notebook_utils.py").exists():
# `notebook_utils` モジュールを取得
import requests
r = requests.get(
url="https://raw.githubusercontent.com/openvinotoolkit/openvino_notebooks/latest/utils/notebook_utils.py",
)
open("notebook_utils.py", "w").write(r.text)
import notebook_utils as utils
from deepsort_utils.tracker import Tracker
from deepsort_utils.nn_matching import NearestNeighborDistanceMetric
from deepsort_utils.detection import (
Detection,
compute_color_for_labels,
xywh_to_xyxy,
xywh_to_tlwh,
tlwh_to_xyxy,
)
モデルのダウンロード#
テストを開始するには、OpenVINO の Open Model Zoo の事前トレーニング済みモデルを使用します。
ここでは、openvino-dev
パッケージのコマンドライン・ツールである omz_downloader
を使用します。ディレクトリー構造が自動的に作成され、選択したモデルがダウンロードされます。モデルがすでにダウンロードされている場合、この手順はスキップされます。選択されたモデルはパブリック・ディレクトリーから取得されます。つまり、OpenVINO 中間表現 (OpenVINO IR) に変換する必要があります。
注: リスト外のモデルを使用する場合は、異なる前処理と後処理が必要になる場合があります。
この場合、人物検出モデルを展開してビデオの各フレーム内の人物を検出し、再識別モデルを使用して、コサイン距離によって人物の画像のペアを一致させる埋め込みベクトルを出力します。
別のモデル (オブジェクト検出モデルリストからの person-detection-xxx
、再識別モデルリストからの person-reidentification-retail-xxx
) をダウンロードする場合は、以下のコードでモデル名を置き換えます。
# モデルがダウンロードされるディレクトリー
base_model_dir = "model"
precision = "FP16"
# The name of the model from Open Model Zoo
detection_model_name = "person-detection-0202"
download_command = (
f"omz_downloader " f"--name {detection_model_name} " f"--precisions {precision} " f"--output_dir {base_model_dir} " f"--cache_dir {base_model_dir}"
)
! $download_command
detection_model_path =
f"model/intel/{detection_model_name}/{precision}/{detection_model_name}.xml"
reidentification_model_name = "person-reidentification-retail-0287"
download_command = (
f"omz_downloader " f"--name {reidentification_model_name} " f"--precisions {precision} " f"--output_dir {base_model_dir} " f"--cache_dir {base_model_dir}"
)
! $download_command
reidentification_model_path =
f"model/intel/{reidentification_model_name}/{precision}/{reidentification_model_name}.xml"
################|| Downloading person-detection-0202 ||################
========== Downloading model/intel/person-detection-0202/FP16/person-detection-0202.xml
========== Downloading model/intel/person-detection-0202/FP16/person-detection-0202.bin
################|| Downloading person-reidentification-retail-0287 ||################
========== Downloading model/intel/person-reidentification-retail-0287/person-reidentification-retail-0267.onnx
========== Downloading model/intel/person-reidentification-retail-0287/FP16/person-reidentification-retail-0287.xml
========== Downloading model/intel/person-reidentification-retail-0287/FP16/person-reidentification-retail-0287.bin
モデルのロード#
モデルの読み込みと予測のための共通クラスを定義します。
OpenVINO モデルの初期化には主に 4 つのステップがあり、推論ループの前に 1 回だけ実行する必要があります。
- OpenVINO ランタイムを初期化します。
*.bin
および*.xml
ファイル (重みとアーキテクチャー) からネットワークを読み取ります。- デバイスのモデルをコンパイルします。
- ノードの入力名と出力名を取得します。
この場合、これらすべてをクラス・コンストラクター関数に配置できます。
OpenVINO が推論に最適なデバイスを自動的に選択するようにするには、AUTO
を使用します。ほとんどの場合、最適なデバイスは GPU
です (パフォーマンスは向上しますが、起動時間がわずかに長くなります)。
core = ov.Core()
class Model:
"""
This class represents a OpenVINO model object.
"""
def __init__(self, model_path, batchsize=1, device="AUTO"):
"""
Initialize the model object
Parameters
----------
model_path: path of inference model
batchsize: batch size of input data
device: device used to run inference
"""
self.model = core.read_model(model=model_path)
self.input_layer = self.model.input(0)
self.input_shape = self.input_layer.shape
self.height = self.input_shape[2]
self.width = self.input_shape[3]
for layer in self.model.inputs:
input_shape = layer.partial_shape
input_shape[0] = batchsize
self.model.reshape({layer: input_shape})
self.compiled_model = core.compile_model(model=self.model, device_name=device)
self.output_layer = self.compiled_model.output(0)
def predict(self, input):
"""
Run inference
Parameters
----------
input: array of input data
"""
result = self.compiled_model(input)[self.output_layer]
return result
推論デバイスの選択#
OpenVINO を使用して推論を実行するためにドロップダウン・リストからデバイスを選択します
import ipywidgets as widgets
device = widgets.Dropdown(
options=core.available_devices + ["AUTO"],
value="AUTO",
description="Device:",
disabled=False,
)
device
Dropdown(description='Device:', index=1, options=('CPU', 'AUTO'), value='AUTO')
detector = Model(detection_model_path, device=device.value)
# 検出オブジェクトの数は不確定であるため、リードモデルの入力バッチサイズは動的であるべきです
extractor = Model(reidentification_model_path, -1, device.value)
データ処理#
データ処理には、データの前処理機能と後処理機能が含まれます。
データ前処理機能は、ネットワーク入力形式の要件に応じて、入力データのレイアウトと形状を変更するために使用されます。
データ後処理機能は、ネットワークの元の出力から有用な情報を抽出し、視覚化するために使用されます。
def preprocess(frame, height, width):
"""
Preprocess a single image
Parameters
----------
frame: input frame
height: height of model input data
width: width of model input data
"""
resized_image = cv2.resize(frame, (width, height))
resized_image = resized_image.transpose((2, 0, 1))
input_image = np.expand_dims(resized_image, axis=0).astype(np.float32)
return input_image
def batch_preprocess(img_crops, height, width):
"""
Preprocess batched images
Parameters
----------
img_crops: batched input images
height: height of model input data
width: width of model input data
"""
img_batch = np.concatenate([preprocess(img, height, width) for img in img_crops], axis=0)
return img_batch
def process_results(h, w, results, thresh=0.5):
"""
postprocess detection results
Parameters
----------
h, w: original height and width of input image
results: raw detection network output
thresh: threshold for low confidence filtering
"""
# 'results' 変数は [1, 1, N, 7] テンソルです
detections = results.reshape(-1, 7)
boxes = []
labels = []
scores = []
for i, detection in enumerate(detections):
_, label, score, xmin, ymin, xmax, ymax = detection
# 検出されたオブジェクトをフィルター処理
if score > thresh:
# 正規化された座標 [0,1] のボックスからピクセル座標のボックスを作成
boxes.append(
[
(xmin + xmax) / 2 * w,
(ymin + ymax) / 2 * h,
(xmax - xmin) * w,
(ymax - ymin) * h,
]
)
labels.append(int(label))
scores.append(float(score))
if len(boxes) == 0:
boxes = np.array([]).reshape(0, 4)
scores = np.array([])
labels = np.array([])
return np.array(boxes), np.array(scores), np.array(labels)
def draw_boxes(img, bbox, identities=None):
"""
Draw bounding box in original image
Parameters
----------
img: original image
bbox: coordinate of bounding box
identities: identities IDs
"""
for i, box in enumerate(bbox):
x1, y1, x2, y2 = [int(i) for i in box]
# ボックステキストとバー
id = int(identities[i]) if identities is not None else 0
color = compute_color_for_labels(id)
label = "{}{:d}".format("", id)
t_size = cv2.getTextSize(label, cv2.FONT_HERSHEY_PLAIN, 2, 2)[0]
cv2.rectangle(img, (x1, y1), (x2, y2), color, 2)
cv2.rectangle(img, (x1, y1), (x1 + t_size[0] + 3, y1 + t_size[1] + 4), color, -1)
cv2.putText(
img,
label,
(x1, y1 + t_size[1] + 4),
cv2.FONT_HERSHEY_PLAIN,
1.6,
[255, 255, 255],
2,
)
return img
def cosin_metric(x1, x2):
"""
Calculate the consin distance of two vector
Parameters
----------
x1, x2: input vectors
"""
return np.dot(x1, x2) / (np.linalg.norm(x1) * np.linalg.norm(x2))
人物再識別モデルのテスト#
再識別ネットワークは、reid_embedding
という名前の (1, 256)
形状のブロブを出力します。これは、コサイン距離を使用して他の記述子と比較できます。
データを可視化#
base_file_link =
"https://storage.openvinotoolkit.org/repositories/openvino_notebooks/data/data/image/person_"
image_indices = ["1_1.png", "1_2.png", "2_1.png"]
image_paths = [utils.download_file(base_file_link + image_index, directory="data") for image_index in image_indices]
image1, image2, image3 = [cv2.cvtColor(cv2.imread(str(image_path)), cv2.COLOR_BGR2RGB) for image_path in image_paths]
# 画像を使用してタイトルを定義
data = {"Person 1": image1, "Person 2": image2, "Person 3": image3}
# 画像を視覚化するサブプロットを作成
fig, axs = plt.subplots(1, len(data.items()), figsize=(5, 5))
# サブプロットを埋める
for ax, (name, image) in zip(axs, data.items()):
ax.axis("off")
ax.set_title(name)
ax.imshow(image)
# 画像を表示
plt.show(fig)
data/person_1_1.png: 0%| | 0.00/68.3k [00:00<?, ?B/s]
data/person_1_2.png: 0%| | 0.00/68.9k [00:00<?, ?B/s]
data/person_2_1.png: 0%| | 0.00/70.3k [00:00<?, ?B/s]
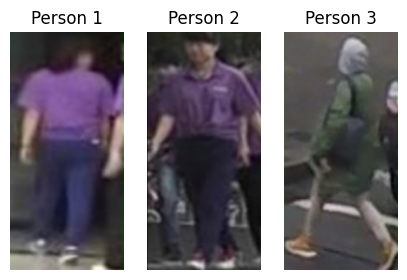
2 人を比較する#
# メトリック・パラメーター
MAX_COSINE_DISTANCE = 0.6 # threshold of matching object
input_data = [image2, image3]
img_batch = batch_preprocess(input_data, extractor.height, extractor.width)
features = extractor.predict(img_batch)
sim = cosin_metric(features[0], features[1])
if sim >= 1 - MAX_COSINE_DISTANCE:
print(f"Same person (confidence: {sim})")
else:
print(f"Different person (confidence: {sim})")
Different person (confidence: 0.02726624347269535)
メイン処理関数#
指定されたソースで人物追跡を実行します。ウェブカメラのフィードまたはビデオファイルのいずれか。
# 人物追跡を実行するメイン処理関数
def run_person_tracking(source=0, flip=False, use_popup=False, skip_first_frames=0):
"""
Main function to run the person tracking:
1. Create a video player to play with target fps (utils.VideoPlayer).
2. Prepare a set of frames for person tracking.
3. Run AI inference for person tracking.
4. Visualize the results.
Parameters:
----------
source: The webcam number to feed the video stream with primary webcam set to "0", or the video path.
flip: To be used by VideoPlayer function for flipping capture image.
use_popup: False for showing encoded frames over this notebook, True for creating a popup window.
skip_first_frames: Number of frames to skip at the beginning of the video.
"""
player = None
try:
# ターゲット fps で再生するビデオプレーヤーを作成
player = utils.VideoPlayer(
source=source,
size=(700, 450),
flip=flip,
fps=24,
skip_first_frames=skip_first_frames,
)
# キャプチャーを開始
player.start()
if use_popup:
title = "Press ESC to Exit"
cv2.namedWindow(winname=title, flags=cv2.WINDOW_GUI_NORMAL | cv2.WINDOW_AUTOSIZE)
processing_times = collections.deque()
while True: # フレームをグラブ
frame = player.next()
if frame is None:
print("Source ended")
break
# フレームがフル HD より大きい場合は、サイズを縮小してパフォーマンスを向上させます
# ニューラル・ネットワークの入力に合わせて画像のサイズを変更し、暗さを変更
h, w = frame.shape[:2]
input_image = preprocess(frame, detector.height, detector.width)
# 処理時間を測定
start_time = time.time()
# Get the results.
output = detector.predict(input_image)
stop_time = time.time()
processing_times.append(stop_time - start_time)
if len(processing_times) > 200:
processing_times.popleft()
_, f_width = frame.shape[:2]
# 平均処理時間 [ms]
processing_time = np.mean(processing_times) * 1100
fps = 1000 / processing_time
# 検出結果からポーズを取得
bbox_xywh, score, label = process_results(h, w, results=output)
img_crops = []
for box in bbox_xywh:
x1, y1, x2, y2 = xywh_to_xyxy(box, h, w)
img = frame[y1:y2, x1:x2]
img_crops.append(img)
# 各人の再識別機能を取得
if img_crops:
# 前処理
img_batch = batch_preprocess(img_crops, extractor.height, extractor.width)
features = extractor.predict(img_batch)
else:
features = np.array([])
# 検出結果と再識別結果をまとめる
bbox_tlwh = xywh_to_tlwh(bbox_xywh)
detections = [Detection(bbox_tlwh[i], features[i]) for i in range(features.shape[0])]
# 追跡対象の位置を予測
tracker.predict()
# トラッカーを更新
tracker.update(detections)
# bbox ID を更新
outputs = []
for track in tracker.tracks:
if not track.is_confirmed() or track.time_since_update > 1:
continue
box = track.to_tlwh()
x1, y1, x2, y2 = tlwh_to_xyxy(box, h, w)
track_id = track.track_id
outputs.append(np.array([x1, y1, x2, y2, track_id], dtype=np.int32))
if len(outputs) > 0:
outputs = np.stack(outputs, axis=0)
# 視覚化のための描画ボックス
if len(outputs) > 0:
bbox_tlwh = []
bbox_xyxy = outputs[:, :4]
identities = outputs[:, -1]
frame = draw_boxes(frame, bbox_xyxy, identities)
cv2.putText(
img=frame,
text=f"Inference time: {processing_time:.1f}ms ({fps:.1f} FPS)",
org=(20, 40),
fontFace=cv2.FONT_HERSHEY_COMPLEX,
fontScale=f_width / 1000,
color=(0, 0, 255),
thickness=1,
lineType=cv2.LINE_AA,
)
if use_popup:
cv2.imshow(winname=title, mat=frame)
key = cv2.waitKey(1)
# escape = 27
if key == 27:
break
else:
# numpy 配列を jpg にエンコード
_, encoded_img = cv2.imencode(ext=".jpg", img=frame, params=[cv2.IMWRITE_JPEG_QUALITY, 100])
# IPython イメージを作成
i = display.Image(data=encoded_img)
# このノートブックに画像を表示
display.clear_output(wait=True)
display.display(i)
# ctrl-c
except KeyboardInterrupt:
print("Interrupted")
# 異なるエラー
except RuntimeError as e:
print(e)
finally:
if player is not None:
# キャプチャーを停止
Player.stop().stop()
if use_popup:
cv2.destroyAllWindows()
実行#
トラッカーを初期化#
新しい追跡タスクを実行する前に、トラッカー・オブジェクトを再初期化する必要があります。
NN_BUDGET = 100
MAX_COSINE_DISTANCE = 0.6 # 一致するオブジェクトのしきい値
metric = NearestNeighborDistanceMetric("cosine", MAX_COSINE_DISTANCE, NN_BUDGET)
tracker = Tracker(metric, max_iou_distance=0.7, max_age=70, n_init=3)
ライブ人物追跡を実行#
Web カメラをビデオ入力として使用します。デフォルトでは、プライマリー Web カメラは source=0
に設定されます。複数のウェブカメラがある場合、0 から始まる連続した番号が割り当てられます。前面カメラを使用する場合は、flip=True
を設定します。一部のウェブブラウザー、特に Mozilla Firefox ではちらつきが発生する場合があります。ちらつきが発生する場合、use_popup=True
を設定してください。
ウェブカメラがない場合でも、ビデオファイルを使用してこのデモを実行できます。OpenCV でサポートされている形式であればどれでも機能します。
USE_WEBCAM = False
cam_id = 0
video_file =
"https://storage.openvinotoolkit.org/repositories/openvino_notebooks/data/data/video/people.mp4"
source = cam_id if USE_WEBCAM else video_file
run_person_tracking(source=source, flip=USE_WEBCAM, use_popup=False)
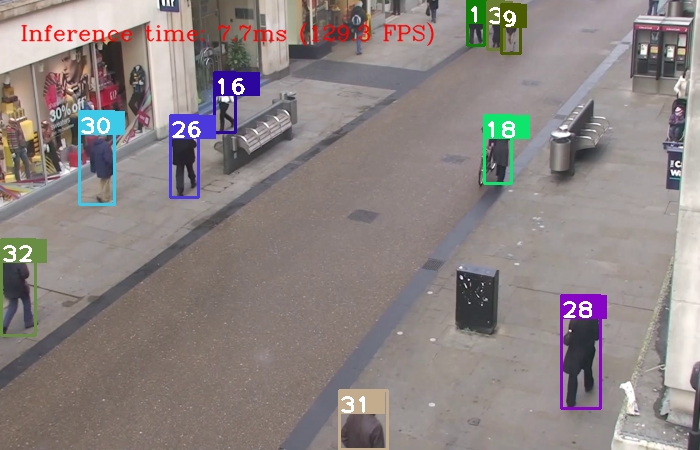
ソースの終わり